Introduction to Python
Course
1761 Learners Already enrolled4.7
(262)
- Grasp the fundamental concepts of Python, including variables, types, and conditional statements.
- Understand complex data types such as lists, tuples, and dictionaries and their associated methods.
- Get acquainted with loops for iteratively handling tasks and nested loops for more complex scenarios.
- Develop proficiency in defining, modifying, and utilizing functions.
Trusted by employees of leading companies
Share it on social media and in your performance review
There are 6 modules in this course
Python is a high-level, interpreted, general-purpose programming language. Distinguished from languages such as HTML, CSS, and JavaScript, which are mainly utilized in web development, Python boasts versatility across multiple domains, including software development, data science, and back-end development. This course will guide you through Python's fundamental concepts, equipping you with the skills to create your own functions by the conclusion of the program.Chosen by students of top schools
Why people choose Codefinity for their career
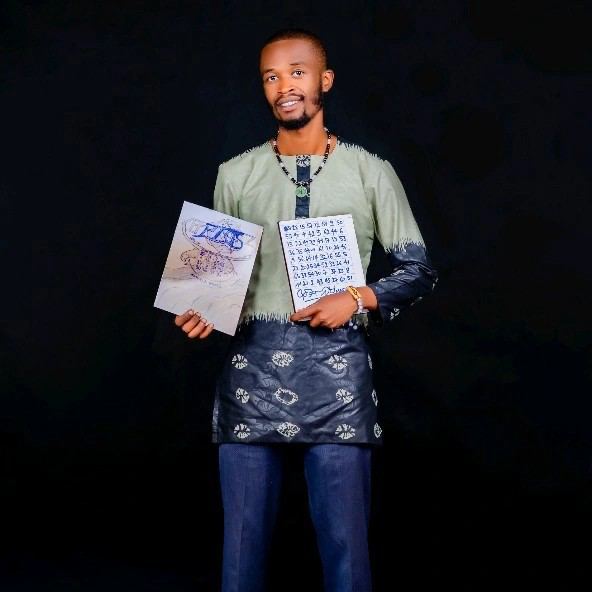
Kwizera Mugisha
The teaching methodology at Codefinity is excellent, and I particularly appreciate how it has prepared me to handle real-world coding problems. Currently, I am delving into Node.js and eagerly anticipate building full-stack projects that integrate all the knowledge I have gained.
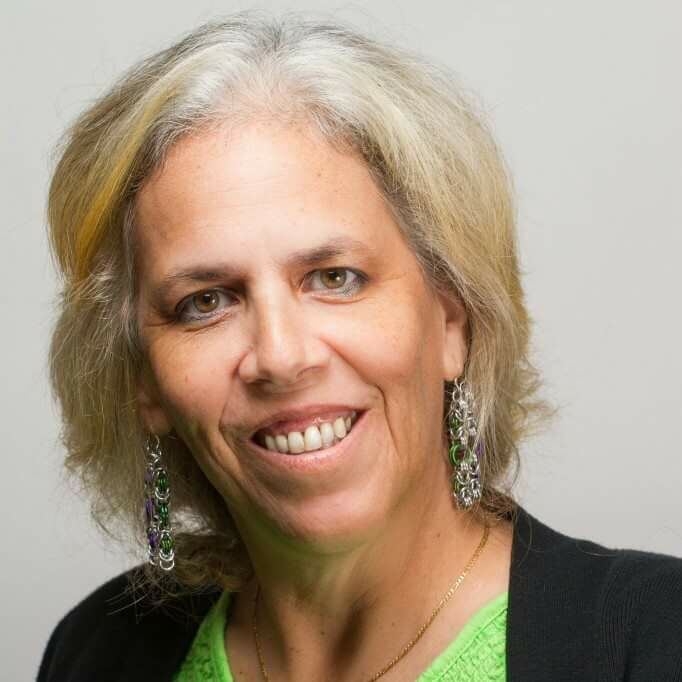
Sherry Barnes-Fox
My first course was 4 hours, I did it in a few days, "nugget-style. The instructions are very clear and easy to understand. There is even a hint to help you get the answer, and if you still cannot get the answer, then you can display the answer. I love the learning style that is used, it engages me.
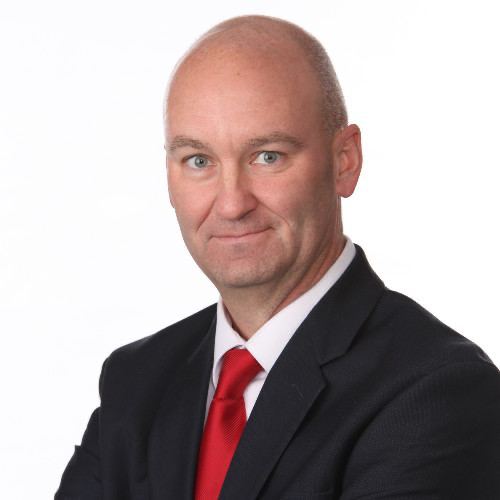
Bill Wagner
I have really liked the browser-based lessons that allow me to code within the lesson. The RUN button allows me to test the code I write before submitting for a grade.
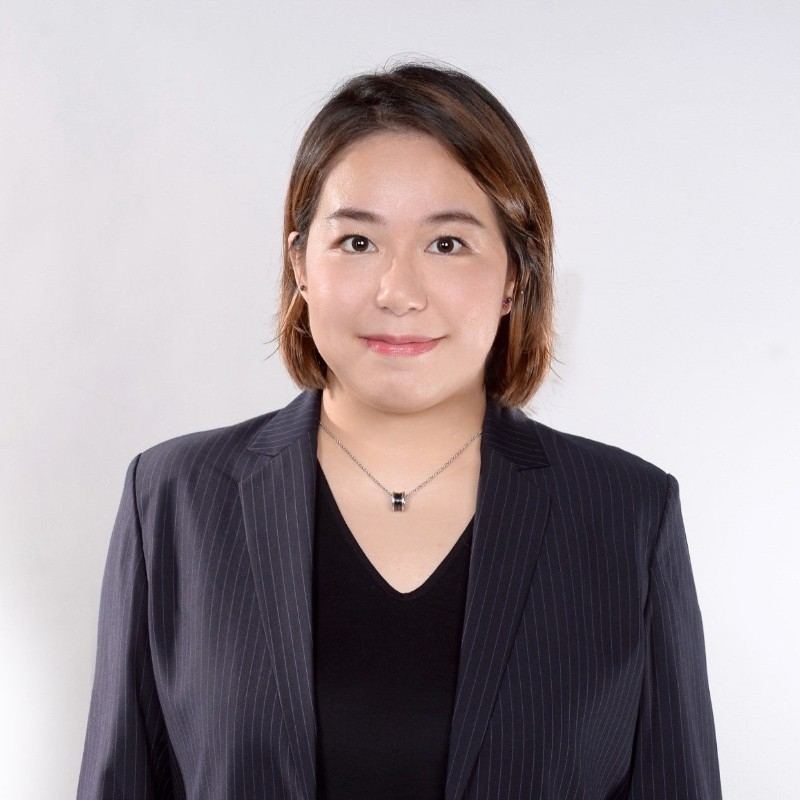
Stephanie Chan
As I went through the first course of the Python track, I liked the way the course was lay out (in easy and digestible modules) with little exercises at the end of each concept.
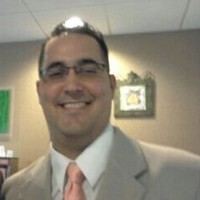
Daniel Chinea
I have gained a lot of practical and logical thinking skills, along with patience for myself and confidence in myself that I can learn programming.
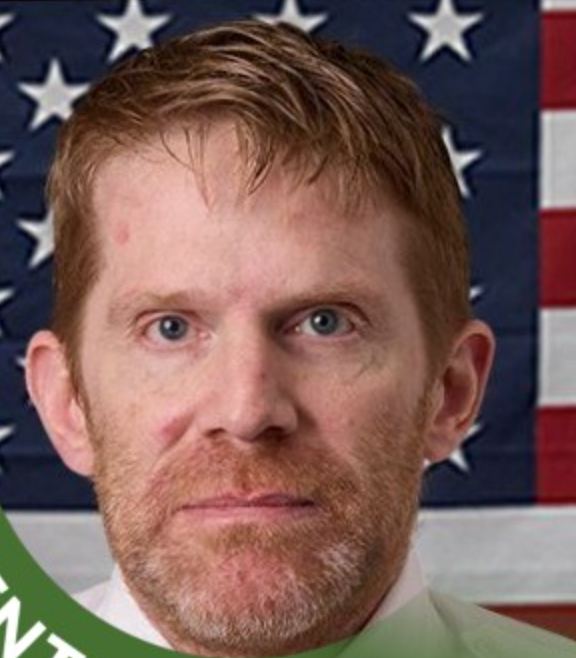
Steve Bruening
The learning was progressive and made it easy to follow along and make progress. I could feel my skills increasing and building on each other as the course went along.
Learner reviews
4.7
50 reviews
5
76%
4
20%
3
4%
2
0%
1
0%
Showing 3 of 50 reviews
Roman Dmytrynskyi
4
Reviewed on Jun 16, 2025
Danylo Nechyporchuk
5
Reviewed on Mar 12, 2025
super
Антон Маринич
4
Reviewed on Feb 28, 2025
Recommended if you're interested in learning Python
Embrace the fascination of Tech Skills! Our AI-assistant provides real-time feedback, personalized hints, and error explanations, empowering you to learn with confidence.
With Workspaces, you can create and share projects directly on our platform. We've prepared templates for your convenience
Take control of your career development and commence your path into mastering the latest technologies
Real-world projects elevate your portfolio, showcasing practical skills to impress potential employers
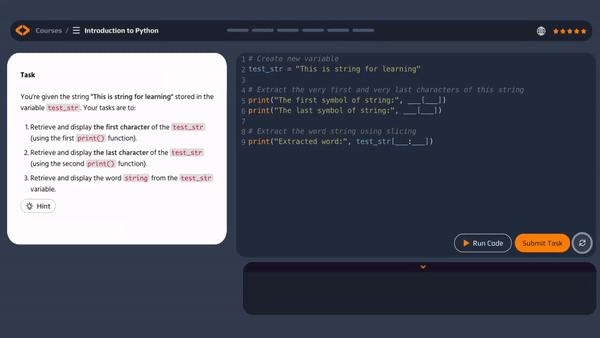
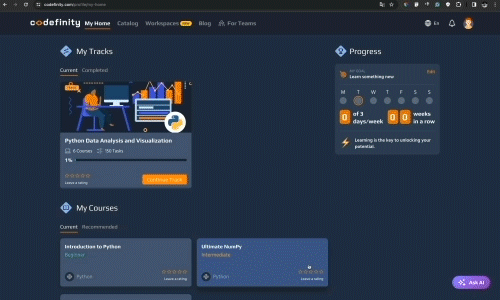
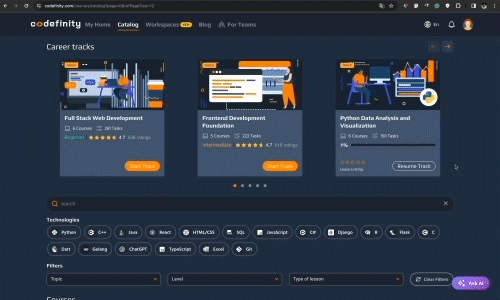
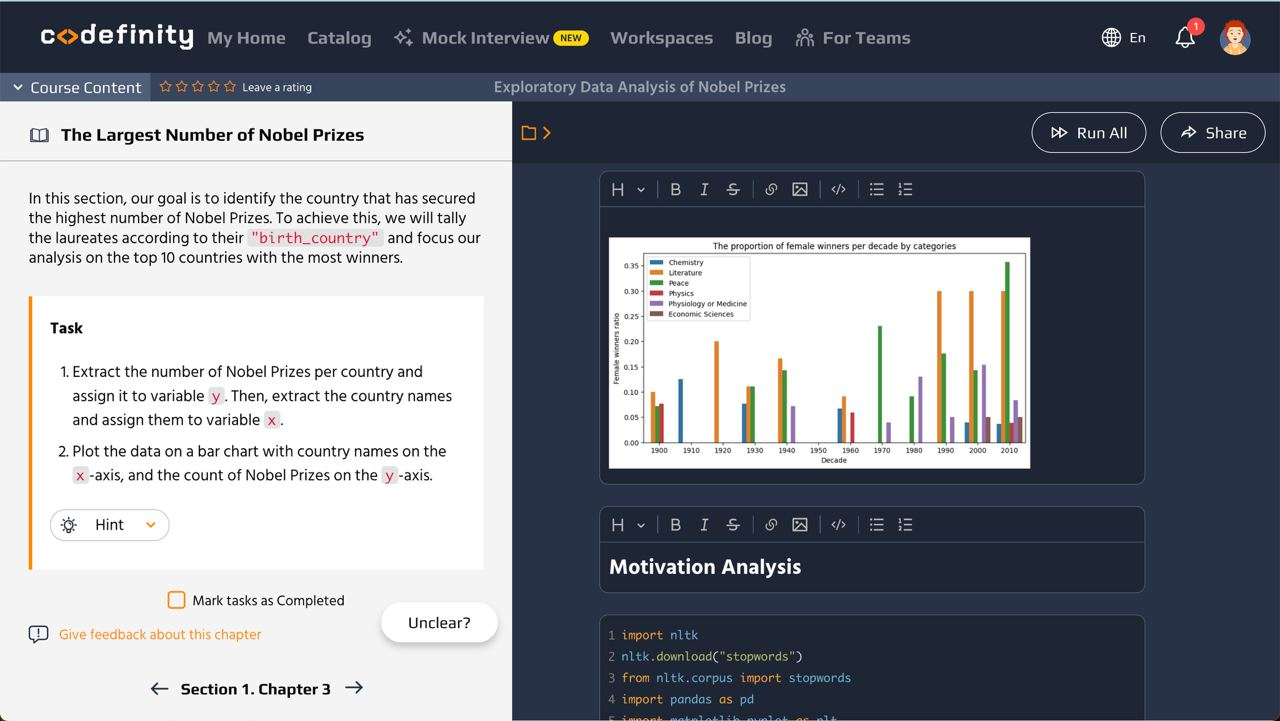
Full catalog access
One subscription opens up this course and our entire catalog of projects and skills.Your subscription also includes:
Frequently asked questions
Still have questions?
Write your question here