Introducción a Python
Curso
1770 Estudiantes ya inscritos4.7
(262)
- Conceptos fundamentales de Python, incluyendo variables, tipos y sentencias condicionales.
- Tipos de datos complejos como listas, tuplas y diccionarios, junto con sus métodos asociados.
- Uso de bucles para la gestión iterativa de tareas y bucles anidados para escenarios más complejos.
- Dominio en la definición, modificación y utilización de funciones.
Confiado por empleados de empresas líderes
Compártelo en redes sociales y en tu evaluación de desempeño
Hay 6 módulos en este curso
Python es un lenguaje de programación de alto nivel, interpretado y de propósito general. A diferencia de lenguajes como HTML, CSS y JavaScript, que se utilizan principalmente en el desarrollo web, Python destaca por su versatilidad en múltiples dominios, incluyendo desarrollo de software, ciencia de datos y desarrollo back-end. Este curso te guiará a través de los conceptos fundamentales de Python, proporcionándote las habilidades necesarias para crear tus propias funciones al finalizar el programa.- Tipos de DatosVista previa
- Almacenamiento de Datos en VariablesVista previa
- Reglas de Nomenclatura de VariablesVista previa
- Uso de VariablesVista previa
- Indexación de Cadenas y LongitudVista previa
- Segmentación y Concatenación de CadenasVista previa
- Desafío: Proyecto Final de Ordenación de InventarioVista previa
- ResumenVista previa
- Listas y Métodos de ListasVista previa
- Listas AnidadasVista previa
- Desafío: Gestión de ListasVista previa
- Tuplas y Métodos de TuplasVista previa
- Operaciones con TuplasVista previa
- Desafío: Operaciones con TuplasVista previa
- Diccionarios y Métodos de DiccionarioVista previa
- Desafío: Proyecto Final de Ajuste de PreciosVista previa
- ResumenVista previa
- Bucles ForVista previa
- Bucles WhileVista previa
- Función RangeVista previa
- Iteración Sobre ÍndicesVista previa
- Desafío: Ventas y EnvíosVista previa
- Bucles AnidadosVista previa
- Desafío: Automatización del Control de InventarioVista previa
- Desafío: Proyecto Final de Codificación IndividualVista previa
- ResumenVista previa
- Funciones IntegradasVista previa
- Desafío: Funciones Zip y SortVista previa
- Funciones Definidas por el UsuarioVista previa
- Comportamiento de Listas en FuncionesVista previa
- Funciones Sin Valor de RetornoVista previa
- Modificación de FuncionesVista previa
- Desafío: Proyecto Final de Ingresos por ProductoVista previa
- ResumenVista previa
Elegido por estudiantes de las mejores escuelas
Por qué la gente elige Codefinity para su carrera
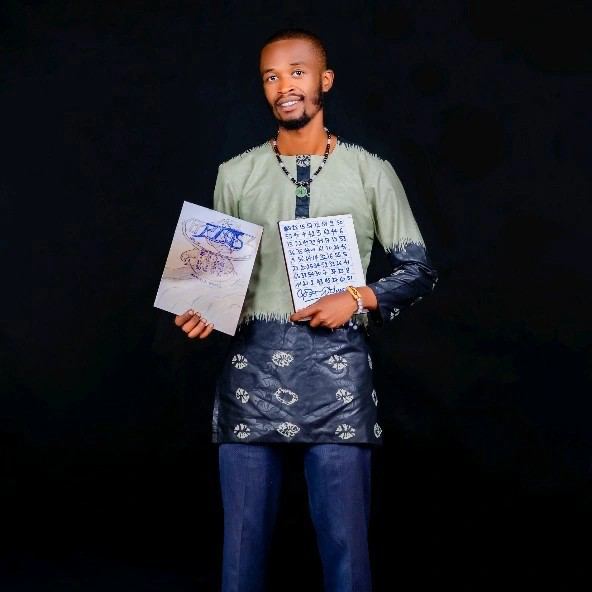
Kwizera Mugisha
The teaching methodology at Codefinity is excellent, and I particularly appreciate how it has prepared me to handle real-world coding problems. Currently, I am delving into Node.js and eagerly anticipate building full-stack projects that integrate all the knowledge I have gained.
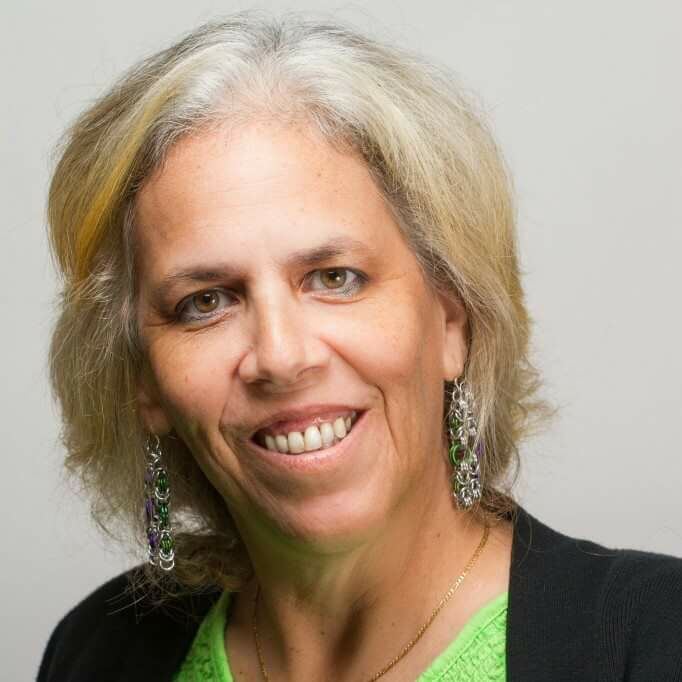
Sherry Barnes-Fox
My first course was 4 hours, I did it in a few days, "nugget-style. The instructions are very clear and easy to understand. There is even a hint to help you get the answer, and if you still cannot get the answer, then you can display the answer. I love the learning style that is used, it engages me.
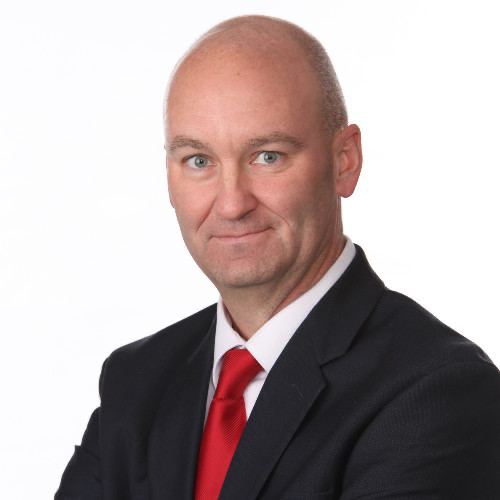
Bill Wagner
I have really liked the browser-based lessons that allow me to code within the lesson. The RUN button allows me to test the code I write before submitting for a grade.
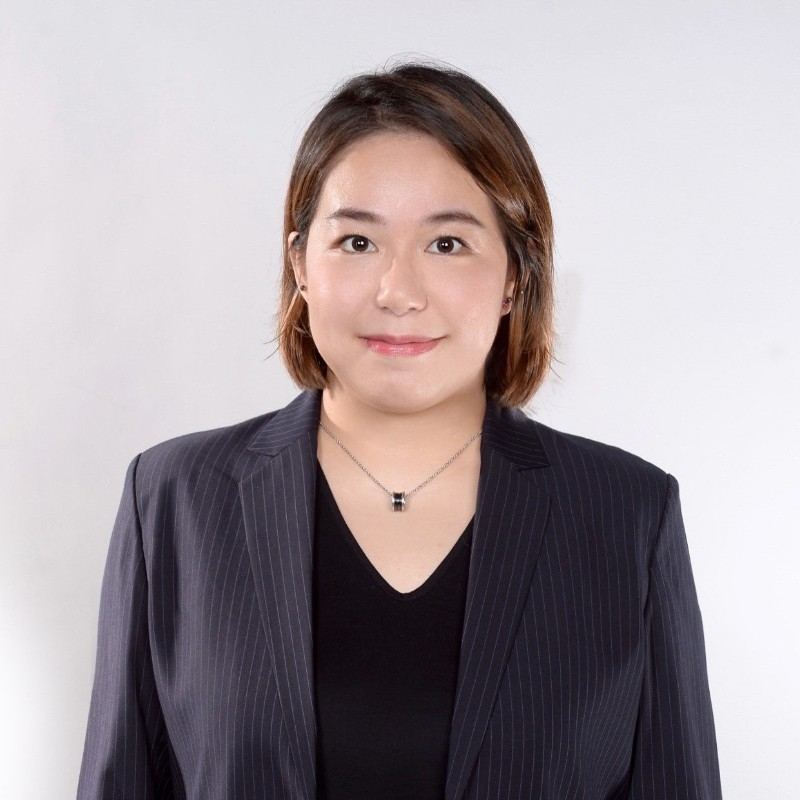
Stephanie Chan
As I went through the first course of the Python track, I liked the way the course was lay out (in easy and digestible modules) with little exercises at the end of each concept.
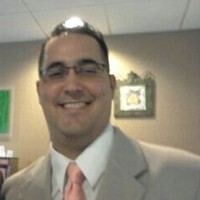
Daniel Chinea
I have gained a lot of practical and logical thinking skills, along with patience for myself and confidence in myself that I can learn programming.
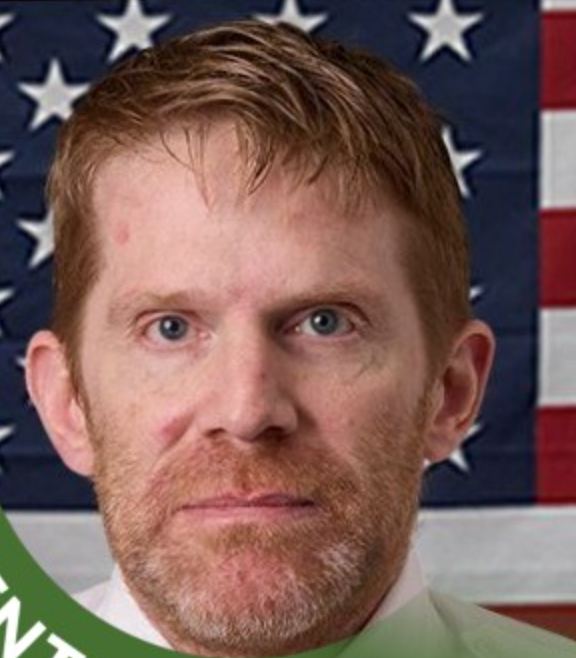
Steve Bruening
The learning was progressive and made it easy to follow along and make progress. I could feel my skills increasing and building on each other as the course went along.
Reseñas de estudiantes
4.7
50 reseñas
5
76%
4
20%
3
4%
2
0%
1
0%
Mostrando 3 de 50 reseñas
Roman Dmytrynskyi
4
Revisado en Jun 16, 2025
Danylo Nechyporchuk
5
Revisado en Mar 12, 2025
super
Антон Маринич
4
Revisado en Feb 28, 2025
Recomendado si estás interesado en aprender Python
curso
Introducción a Python
curso
Introducción a Python
curso
Introducción a Python
curso
Introducción a Python
curso
Introducción a Python
curso
TEST FREE COURSE
curso
Introducción a C++
curso
Conceptos básicos de C#
proyecto
Building a Classic Snake Game
curso
Fundamentos de C
proyecto
Building a Classic Snake Game
curso
C Básico
curso
Introducción a Python
curso
Introducción a Python
curso
Introducción a Python
curso
Introducción a Python
curso
Introducción a Python
curso
TEST FREE COURSE
curso
Introducción a C++
curso
Conceptos básicos de C#
proyecto
Building a Classic Snake Game
curso
Fundamentos de C
proyecto
Building a Classic Snake Game
curso
C Básico
¡Abraza la fascinación de las habilidades tecnológicas! Nuestro asistente de IA proporciona retroalimentación en tiempo real, pistas personalizadas y explicaciones de errores, empoderándote para aprender con confianza.
Con Espacios de trabajo, puedes crear y compartir proyectos directamente en nuestra plataforma. Hemos preparado plantillas para tu conveniencia
Toma el control de tu desarrollo profesional y comienza tu camino hacia el dominio de las últimas tecnologías
Los proyectos del mundo real elevan tu portafolio, mostrando habilidades prácticas para impresionar a posibles empleadores
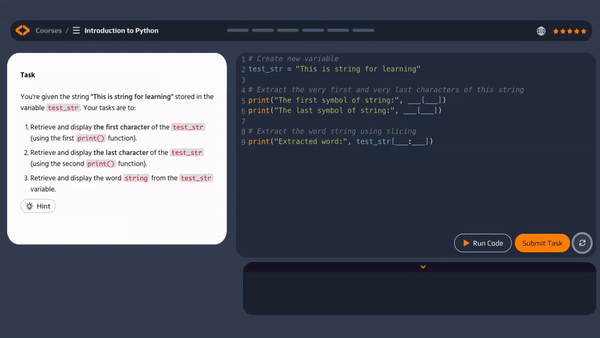
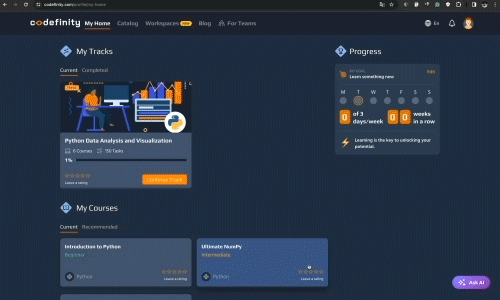
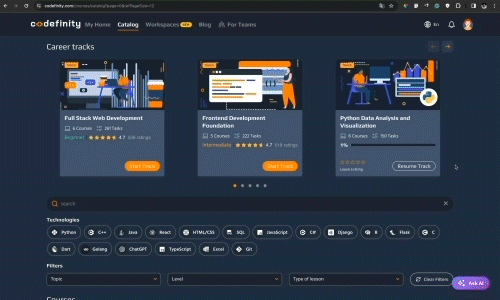
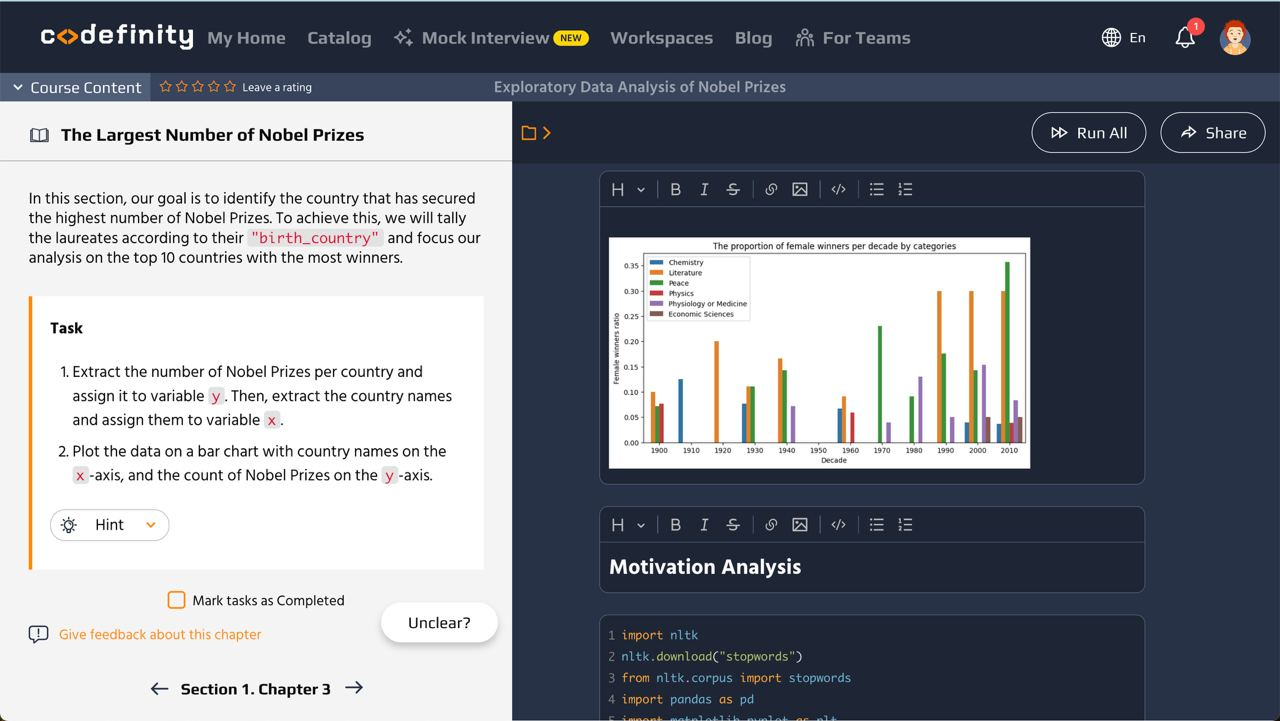
Acceso completo al catálogo
Una suscripción abre este curso y todo nuestro catálogo de proyectos y habilidades.Tu suscripción también incluye:
curso
Introducción a Python
curso
Introducción a Python
curso
Introducción a Python
curso
Introducción a Python
curso
Introducción a Python
curso
TEST FREE COURSE
curso
Introducción a C++
curso
Conceptos básicos de C#
proyecto
Building a Classic Snake Game
curso
Fundamentos de C
proyecto
Building a Classic Snake Game
curso
C Básico
curso
Introducción a Python
curso
Introducción a Python
curso
Introducción a Python
curso
Introducción a Python
curso
Introducción a Python
curso
TEST FREE COURSE
curso
Introducción a C++
curso
Conceptos básicos de C#
proyecto
Building a Classic Snake Game
curso
Fundamentos de C
proyecto
Building a Classic Snake Game
curso
C Básico
Preguntas frecuentes
¿Aún tienes preguntas?
Escribe tu pregunta aquí