Course Content
C++ Introduction
4. Introduction to Program Flow
5. Introduction to Functions
C++ Introduction
Do...while Loop
Unlike a while
loop, which may never execute, a do...while loop is guaranteed to execute at least once. Structure of do…while loop:
Note
The line containing the while part ends with a semicolon (
;
)
Now let's compare the while and do…while loops.
The while loop:
main.cpp
The do...while loop:
main.cpp
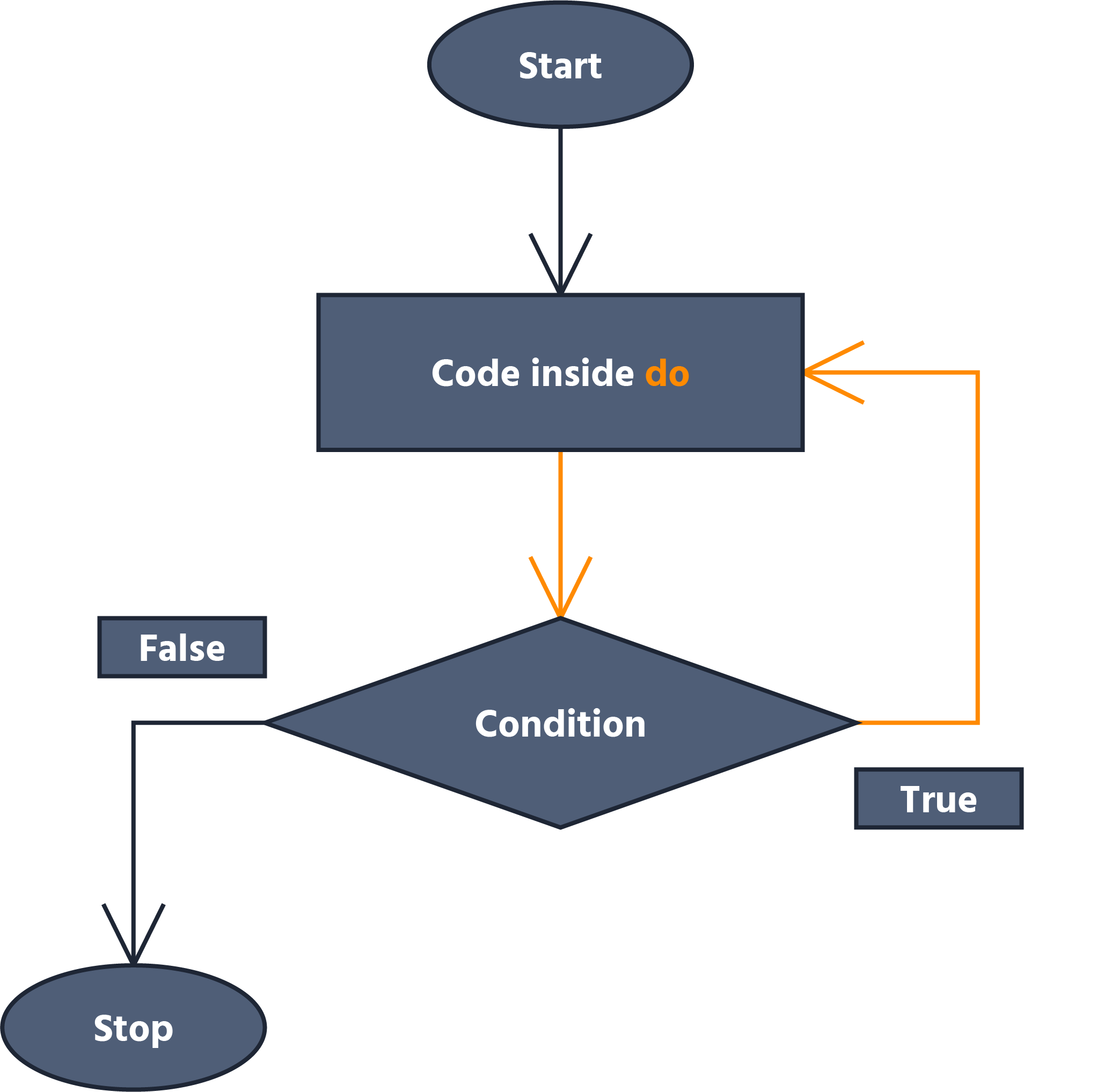
The do...while loop executed one time when the while loop would never have executed.
Everything was clear?
Course Content
C++ Introduction
4. Introduction to Program Flow
5. Introduction to Functions
C++ Introduction
Do...while Loop
Unlike a while
loop, which may never execute, a do...while loop is guaranteed to execute at least once. Structure of do…while loop:
Note
The line containing the while part ends with a semicolon (
;
)
Now let's compare the while and do…while loops.
The while loop:
main.cpp
The do...while loop:
main.cpp
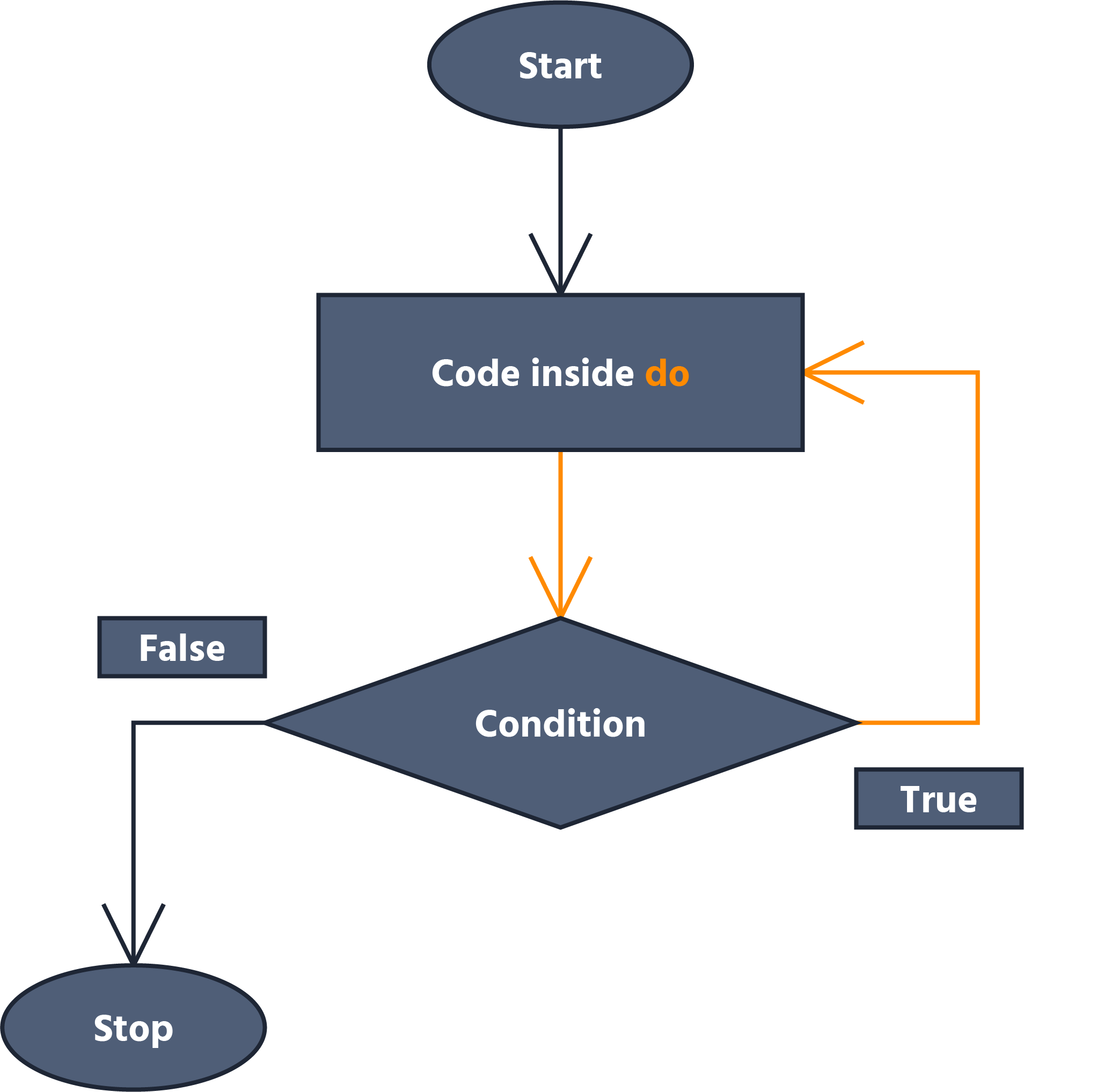
The do...while loop executed one time when the while loop would never have executed.
Everything was clear?