Вступ до Python
Курс
1769 Вже зареєстровані учні4.7
(262)
- Опанування основних понять Python, зокрема змінних, типів та умовних операторів.
- Розуміння складних типів даних, таких як списки, кортежі та словники, а також їхніх методів.
- Ознайомлення з циклами для ітеративної обробки завдань і вкладеними циклами для складніших сценаріїв.
- Формування навичок визначення, модифікації та використання функцій.
Довіряють працівники провідних компаній
Поділіться цим у соціальних мережах та у своєму огляді продуктивності
Є 6 модулі в цьому курсі
Python — це мова програмування високого рівня з інтерпретатором загального призначення. На відміну від таких мов, як HTML, CSS та JavaScript, які переважно використовуються у веброзробці, Python вирізняється універсальністю та застосовується у багатьох сферах, зокрема у розробці програмного забезпечення, науці про дані та бекенд-розробці. Цей курс ознайомить вас з основними поняттями Python і надасть навички для створення власних функцій до завершення програми.- Типи ДанихПопередній перегляд
- Збереження Даних у ЗміннихПопередній перегляд
- Правила Іменування ЗміннихПопередній перегляд
- Використання ЗміннихПопередній перегляд
- Індексація Рядків та ДовжинаПопередній перегляд
- Нарізання та конкатенація РядківПопередній перегляд
- Завдання: Підсумковий Проєкт Із Сортування ІнвентарюПопередній перегляд
- ПідсумокПопередній перегляд
- Логічний Тип ДанихПопередній перегляд
- Комбінування УмовПопередній перегляд
- Завдання: Булева ЛогікаПопередній перегляд
- Оператори Належності та Порівняння ТипівПопередній перегляд
- Умовні ВиразиПопередній перегляд
- Челендж: Підсумковий Проєкт з Управління ПродуктомПопередній перегляд
- ПідсумокПопередній перегляд
- Списки та методи списківПопередній перегляд
- Вкладені СпискиПопередній перегляд
- Завдання: Керування СпискамиПопередній перегляд
- Кортежі та методи кортежівПопередній перегляд
- Операції з КортежамиПопередній перегляд
- Завдання: Операції з КортежамиПопередній перегляд
- Словники та методи словниківПопередній перегляд
- Завдання: Підсумковий Проєкт з Коригування ЦінПопередній перегляд
- ПідсумокПопередній перегляд
- Цикли ForПопередній перегляд
- Цикли WhileПопередній перегляд
- Функція RangeПопередній перегляд
- Ітерація за ІндексамиПопередній перегляд
- Завдання: Продажі та ВідвантаженняПопередній перегляд
- Вкладені ЦиклиПопередній перегляд
- Завдання: Автоматизація Контролю ЗапасівПопередній перегляд
- Виклик: Індивідуальний Підсумковий Проєкт з ПрограмуванняПопередній перегляд
- ПідсумокПопередній перегляд
- Вбудовані ФункціїПопередній перегляд
- Завдання: Функції Zip і SortПопередній перегляд
- Користувацькі ФункціїПопередній перегляд
- Поведінка Списків у ФункціяхПопередній перегляд
- Функції без Повернення ЗначенняПопередній перегляд
- Модифікація ФункційПопередній перегляд
- Завдання: Підсумковий Проєкт з Доходів від ПродуктуПопередній перегляд
- ПідсумокПопередній перегляд
З нами навчаються студенти найкращих університетів світу
Чому люди обирають Codefinity для своєї кар'єри
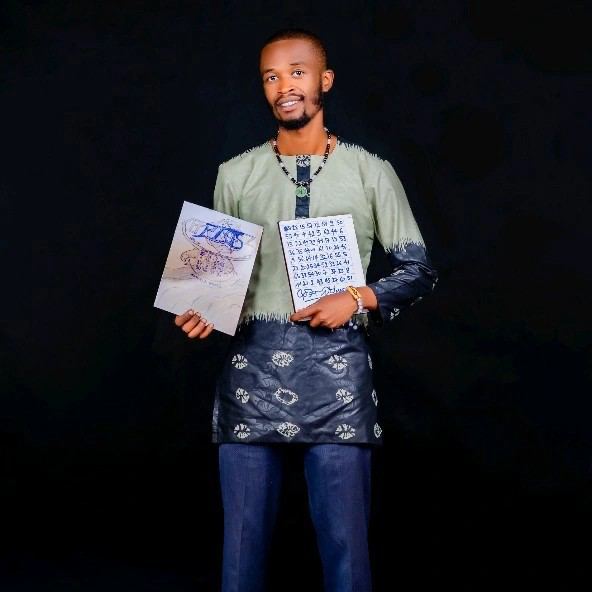
Kwizera Mugisha
The teaching methodology at Codefinity is excellent, and I particularly appreciate how it has prepared me to handle real-world coding problems. Currently, I am delving into Node.js and eagerly anticipate building full-stack projects that integrate all the knowledge I have gained.
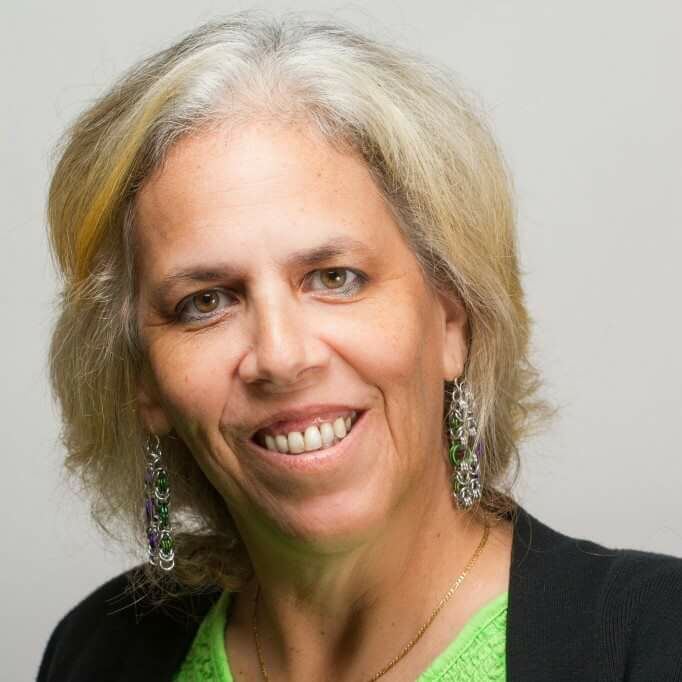
Sherry Barnes-Fox
My first course was 4 hours, I did it in a few days, "nugget-style. The instructions are very clear and easy to understand. There is even a hint to help you get the answer, and if you still cannot get the answer, then you can display the answer. I love the learning style that is used, it engages me.
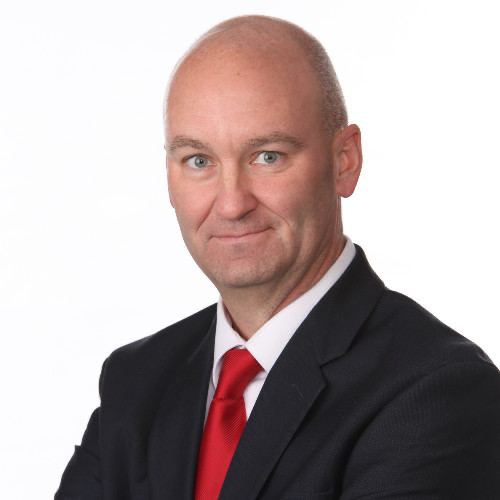
Bill Wagner
I have really liked the browser-based lessons that allow me to code within the lesson. The RUN button allows me to test the code I write before submitting for a grade.
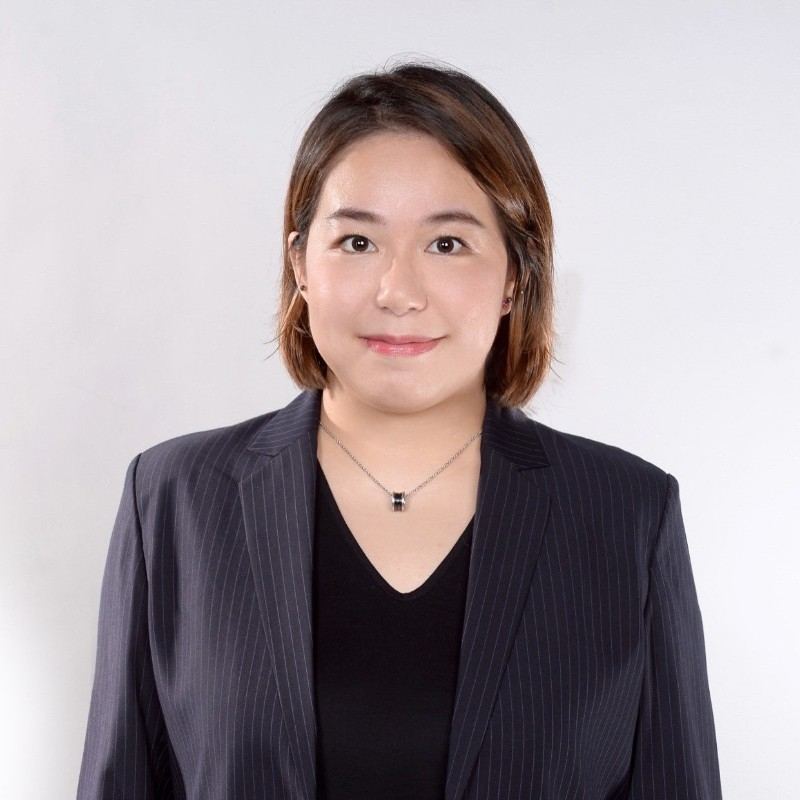
Stephanie Chan
As I went through the first course of the Python track, I liked the way the course was lay out (in easy and digestible modules) with little exercises at the end of each concept.
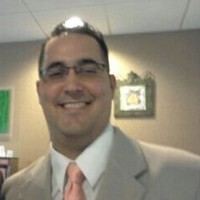
Daniel Chinea
I have gained a lot of practical and logical thinking skills, along with patience for myself and confidence in myself that I can learn programming.
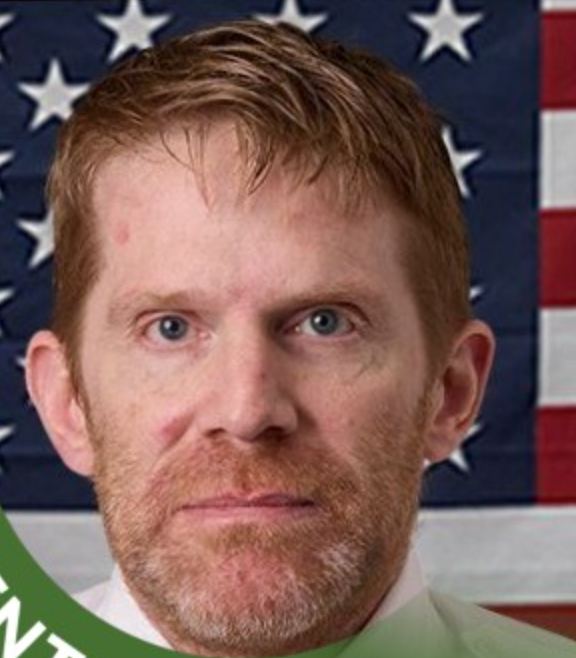
Steve Bruening
The learning was progressive and made it easy to follow along and make progress. I could feel my skills increasing and building on each other as the course went along.
Відгуки учнів
4.7
50 відгуки
5
76%
4
20%
3
4%
2
0%
1
0%
Показано 3 з 50 відгуки
Roman Dmytrynskyi
4
Переглянуто на Jun 16, 2025
Danylo Nechyporchuk
5
Переглянуто на Mar 12, 2025
super
Антон Маринич
4
Переглянуто на Feb 28, 2025
Рекомендовано, якщо ви зацікавлені в навчанні Python
курс
Вступ до Python
курс
Вступ до Python
курс
Вступ до Python
курс
Вступ до Python
курс
Вступ до Python
курс
TEST FREE COURSE
курс
Вступ до C++
курс
Основи C#
проєкт
Building a Classic Snake Game
курс
Основи C
проєкт
Building a Classic Snake Game
курс
Основи C
курс
Вступ до Python
курс
Вступ до Python
курс
Вступ до Python
курс
Вступ до Python
курс
Вступ до Python
курс
TEST FREE COURSE
курс
Вступ до C++
курс
Основи C#
проєкт
Building a Classic Snake Game
курс
Основи C
проєкт
Building a Classic Snake Game
курс
Основи C
Захоплюйтеся технічними навичками! Наш ШІ-асистент надає зворотний зв'язок у реальному часі, персоналізовані підказки та пояснення помилок, надаючи вам можливість вчитися з упевненістю.
Завдяки робочим просторам ви можете створювати та ділитися проектами безпосередньо на нашій платформі. Ми підготували шаблони для вашої зручності
Візьміть під контроль свій кар'єрний розвиток і почніть свій шлях до освоєння новітніх технологій
Проекти з реального світу підвищують ваш портфоліо, демонструючи практичні навички, щоб вразити потенційних роботодавців
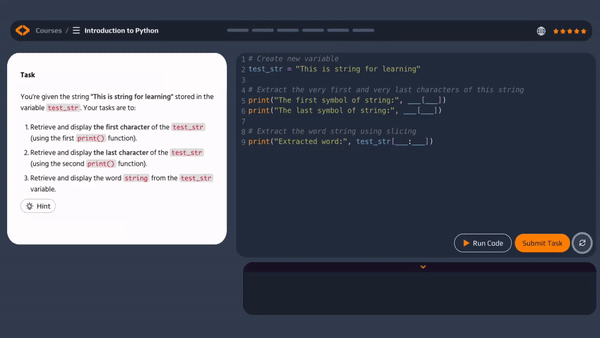
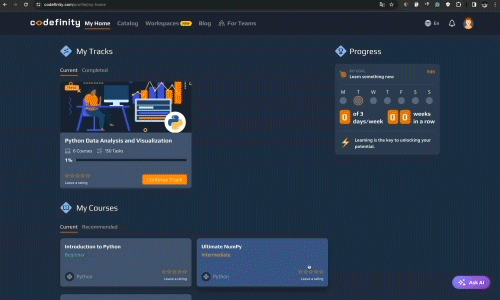
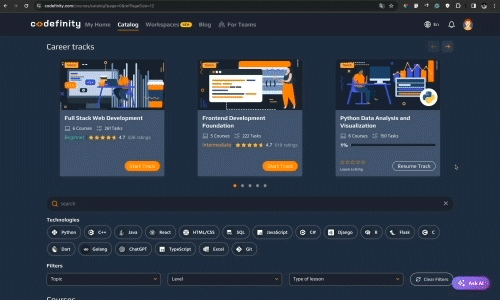
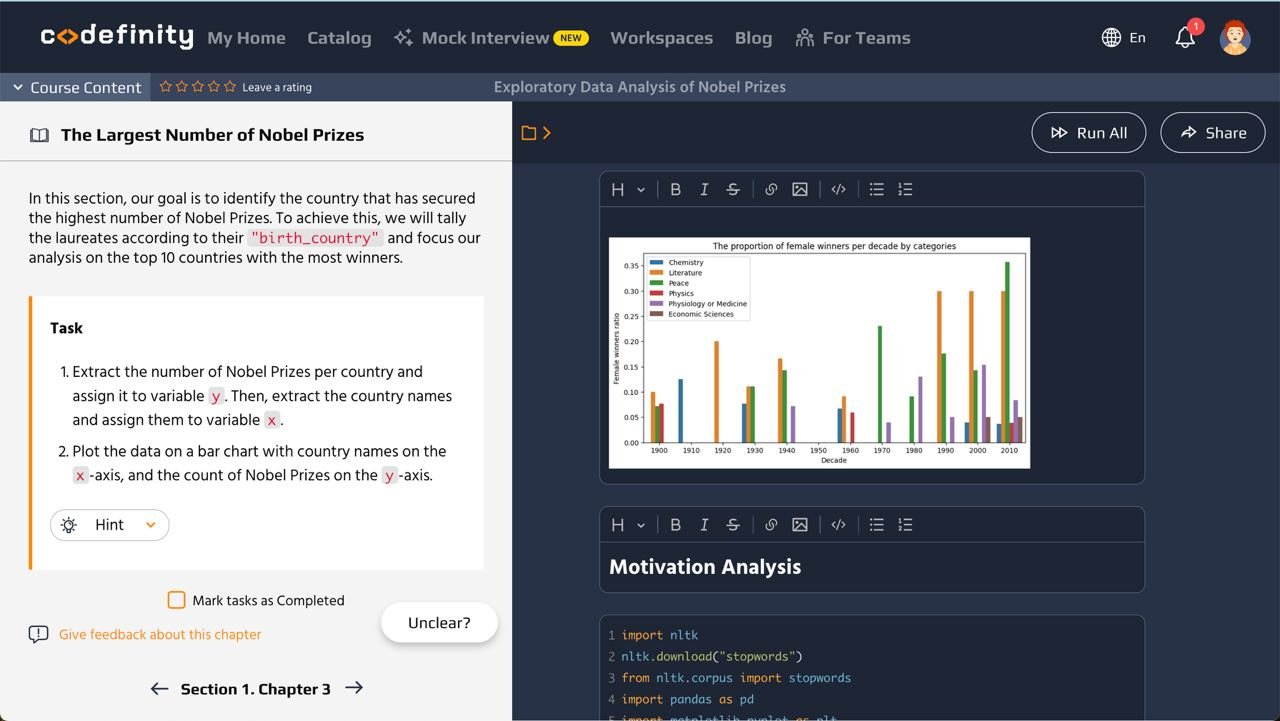
Повний доступ до каталогу
Одна підписка відкриває цей курс і весь наш каталог проектів і навичок.Ваша підписка також включає:
курс
Вступ до Python
курс
Вступ до Python
курс
Вступ до Python
курс
Вступ до Python
курс
Вступ до Python
курс
TEST FREE COURSE
курс
Вступ до C++
курс
Основи C#
проєкт
Building a Classic Snake Game
курс
Основи C
проєкт
Building a Classic Snake Game
курс
Основи C
курс
Вступ до Python
курс
Вступ до Python
курс
Вступ до Python
курс
Вступ до Python
курс
Вступ до Python
курс
TEST FREE COURSE
курс
Вступ до C++
курс
Основи C#
проєкт
Building a Classic Snake Game
курс
Основи C
проєкт
Building a Classic Snake Game
курс
Основи C
Часті запитання
Ще є запитання?
Напишіть ваше запитання тут