Introdução ao Python
Curso
1769 Alunos já inscritos4.7
(262)
- Compreensão dos conceitos fundamentais de Python, incluindo variáveis, tipos e estruturas condicionais.
- Entendimento de tipos de dados complexos, como listas, tuplas e dicionários, bem como de seus métodos associados.
- Familiarização com laços para manipulação iterativa de tarefas e laços aninhados para cenários mais complexos.
- Desenvolvimento de proficiência na definição, modificação e utilização de funções.
Confiado por funcionários de empresas líderes
Compartilhe nas redes sociais e na sua avaliação de desempenho
Existem 6 módulos neste curso
Python é uma linguagem de programação de alto nível, interpretada e de uso geral. Diferenciando-se de linguagens como HTML, CSS e JavaScript, que são utilizadas principalmente no desenvolvimento web, Python destaca-se por sua versatilidade em diversos domínios, incluindo desenvolvimento de software, ciência de dados e desenvolvimento back-end. Este curso irá guiá-lo pelos conceitos fundamentais de Python, capacitando-o a criar suas próprias funções ao final do programa.- Tipos de DadosPré-visualização
- Armazenando Dados em VariáveisPré-visualização
- Regras de Nomenclatura de VariáveisPré-visualização
- Usando VariáveisPré-visualização
- Indexação de Strings e ComprimentoPré-visualização
- Fatiamento e Concatenação de StringsPré-visualização
- Desafio: Projeto Final de Ordenação de InventárioPré-visualização
- RecapitulaçãoPré-visualização
- Tipo de Dado BooleanoPré-visualização
- Combinando CondiçõesPré-visualização
- Desafio: Lógica BooleanaPré-visualização
- Operadores de Associação e Comparações de TipoPré-visualização
- Expressões CondicionaisPré-visualização
- Desafio: Projeto Final de Gestão de ProdutoPré-visualização
- RecapitulaçãoPré-visualização
- Listas e Métodos de ListasPré-visualização
- Listas AninhadasPré-visualização
- Desafio: Gerenciamento de ListasPré-visualização
- Tuplas e Métodos de TuplaPré-visualização
- Operações com TuplasPré-visualização
- Desafio: Operações com TuplasPré-visualização
- Dicionários e Métodos de DicionárioPré-visualização
- Desafio: Projeto Final de Ajuste de PreçosPré-visualização
- RecapitulaçãoPré-visualização
- Laços ForPré-visualização
- Laços WhilePré-visualização
- Função RangePré-visualização
- Iterando Sobre ÍndicesPré-visualização
- Desafio: Vendas e RemessasPré-visualização
- Loops AninhadosPré-visualização
- Desafio: Automatizando o Controle de EstoquePré-visualização
- Desafio: Projeto Final de Codificação IndividualPré-visualização
- RecapitulaçãoPré-visualização
- Funções EmbutidasPré-visualização
- Desafio: Funções Zip e SortPré-visualização
- Funções Definidas pelo UsuárioPré-visualização
- Comportamento de Listas em FunçõesPré-visualização
- Funções sem RetornoPré-visualização
- Modificando FunçõesPré-visualização
- Desafio: Capstone de Receitas de ProdutosPré-visualização
- RecapitulaçãoPré-visualização
Escolhido por estudantes das melhores escolas
Por que as pessoas escolhem o Codefinity para sua carreira
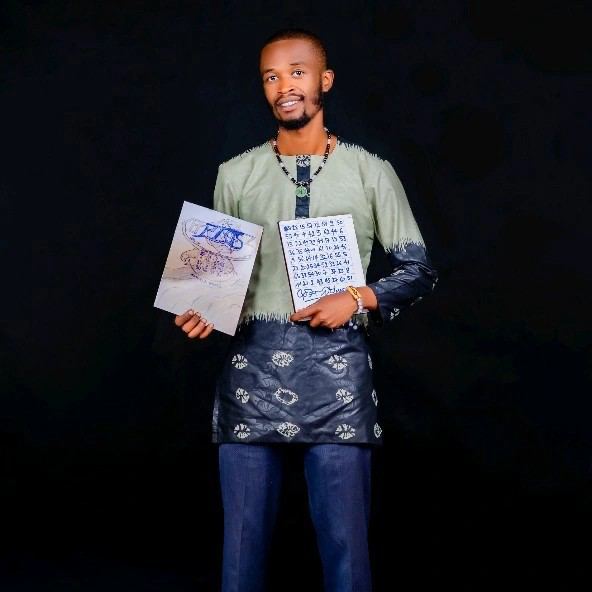
Kwizera Mugisha
The teaching methodology at Codefinity is excellent, and I particularly appreciate how it has prepared me to handle real-world coding problems. Currently, I am delving into Node.js and eagerly anticipate building full-stack projects that integrate all the knowledge I have gained.
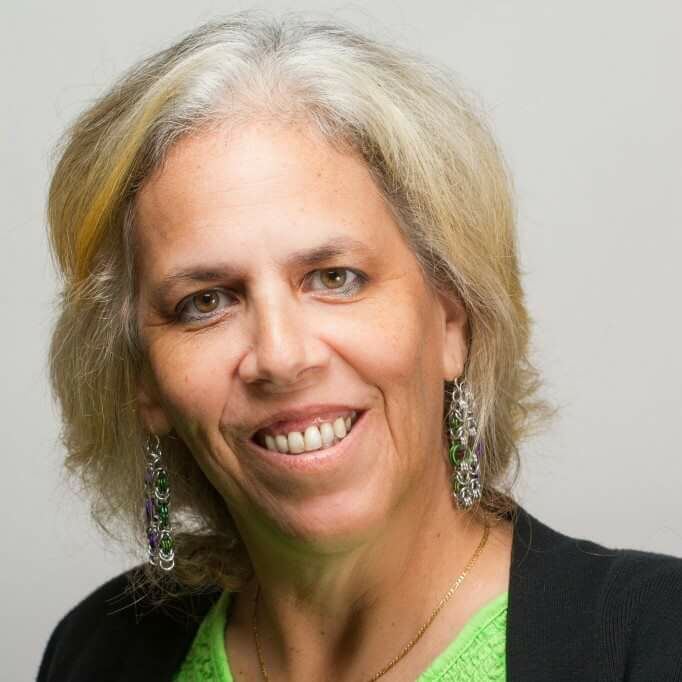
Sherry Barnes-Fox
My first course was 4 hours, I did it in a few days, "nugget-style. The instructions are very clear and easy to understand. There is even a hint to help you get the answer, and if you still cannot get the answer, then you can display the answer. I love the learning style that is used, it engages me.
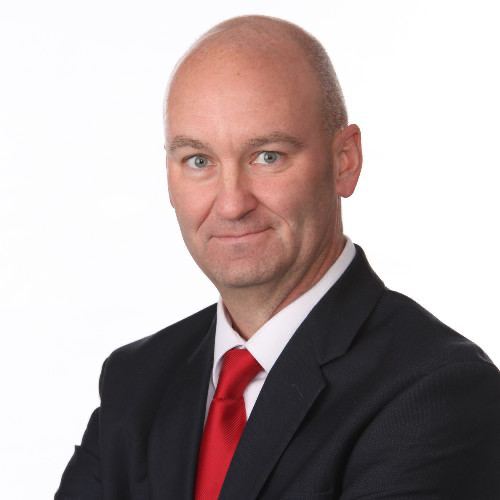
Bill Wagner
I have really liked the browser-based lessons that allow me to code within the lesson. The RUN button allows me to test the code I write before submitting for a grade.
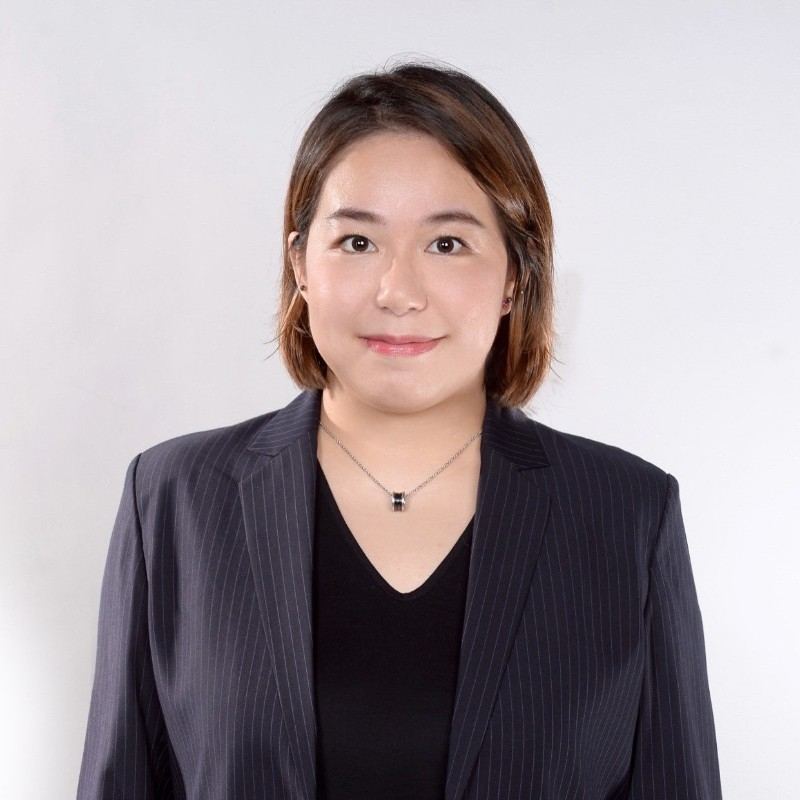
Stephanie Chan
As I went through the first course of the Python track, I liked the way the course was lay out (in easy and digestible modules) with little exercises at the end of each concept.
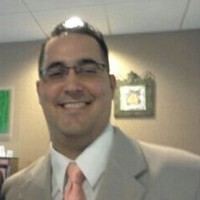
Daniel Chinea
I have gained a lot of practical and logical thinking skills, along with patience for myself and confidence in myself that I can learn programming.
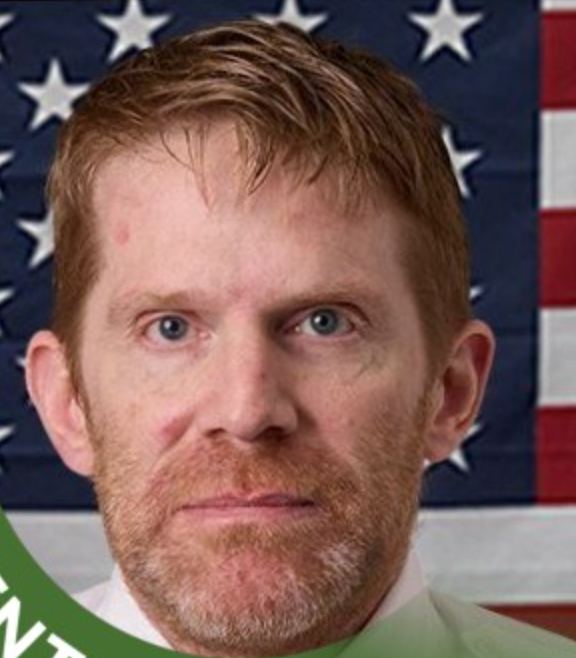
Steve Bruening
The learning was progressive and made it easy to follow along and make progress. I could feel my skills increasing and building on each other as the course went along.
Avaliações de alunos
4.7
50 avaliações
5
76%
4
20%
3
4%
2
0%
1
0%
Mostrando 3 de 50 avaliações
Roman Dmytrynskyi
4
Avaliado em Jun 16, 2025
Danylo Nechyporchuk
5
Avaliado em Mar 12, 2025
super
Антон Маринич
4
Avaliado em Feb 28, 2025
Recomendado se você estiver interessado em aprender Python
curso
Introdução ao Python
curso
Introdução ao Python
curso
Introdução ao Python
curso
Introdução ao Python
curso
Introdução ao Python
curso
TEST FREE COURSE
curso
Introdução ao C++
curso
Noções Básicas de C#
projeto
Building a Classic Snake Game
curso
Fundamentos de C
projeto
Building a Classic Snake Game
curso
Fundamentos de C
curso
Introdução ao Python
curso
Introdução ao Python
curso
Introdução ao Python
curso
Introdução ao Python
curso
Introdução ao Python
curso
TEST FREE COURSE
curso
Introdução ao C++
curso
Noções Básicas de C#
projeto
Building a Classic Snake Game
curso
Fundamentos de C
projeto
Building a Classic Snake Game
curso
Fundamentos de C
Abrace a fascinação das habilidades tecnológicas! Nosso assistente de IA fornece feedback em tempo real, dicas personalizadas e explicações de erros, capacitando você a aprender com confiança.
Com os Espaços de Trabalho, você pode criar e compartilhar projetos diretamente em nossa plataforma. Preparamos modelos para sua conveniência
Assuma o controle do desenvolvimento de sua carreira e comece seu caminho para dominar as tecnologias mais recentes
Projetos do mundo real elevam seu portfólio, mostrando habilidades práticas para impressionar potenciais empregadores
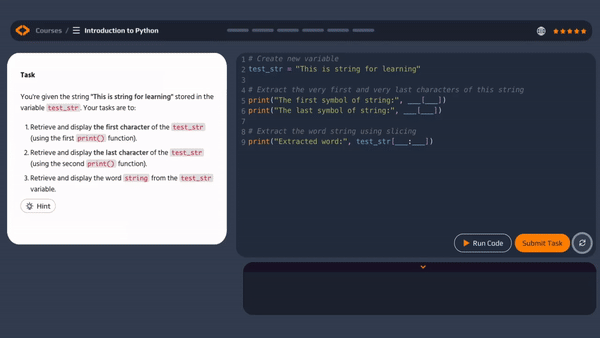
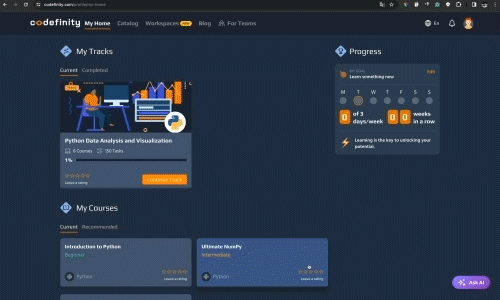
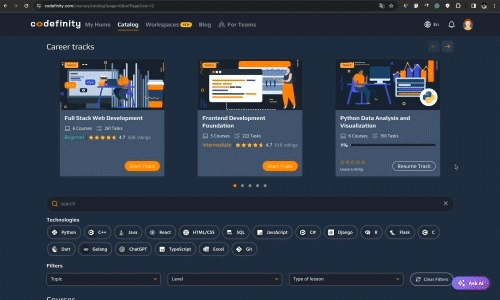
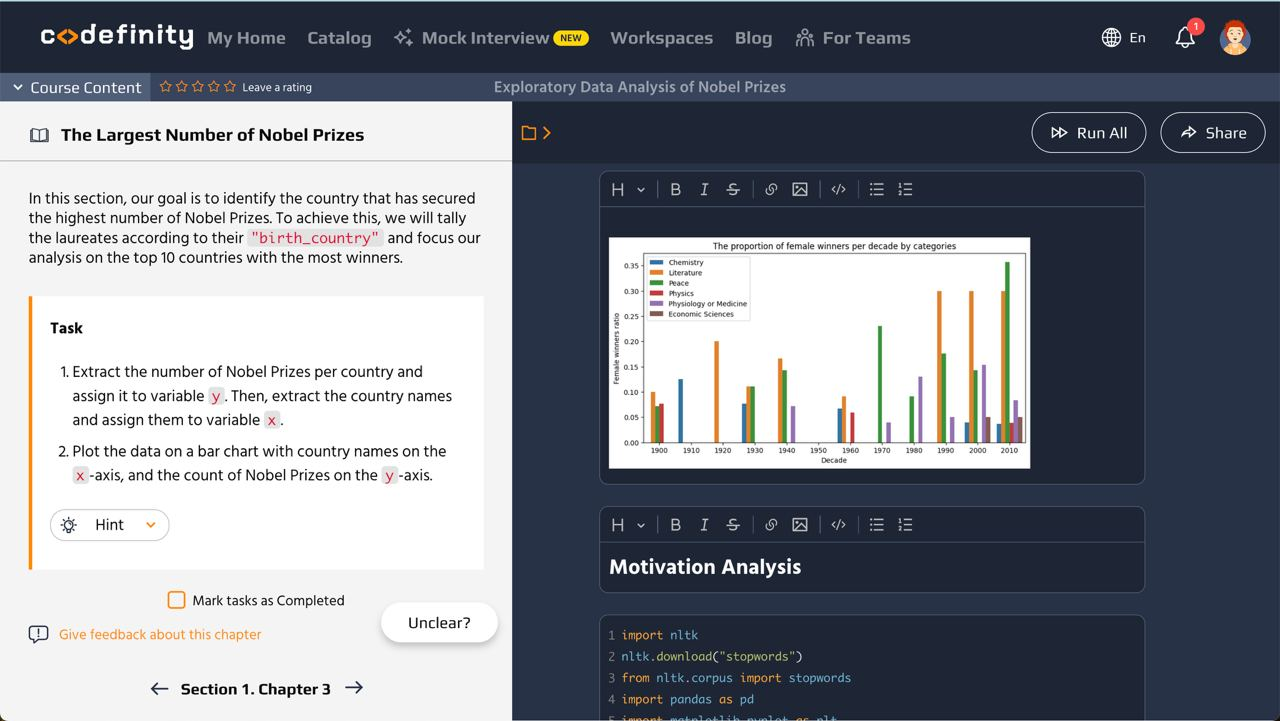
Acesso completo ao catálogo
Uma assinatura abre este curso e todo o nosso catálogo de projetos e habilidades.Sua assinatura também inclui:
curso
Introdução ao Python
curso
Introdução ao Python
curso
Introdução ao Python
curso
Introdução ao Python
curso
Introdução ao Python
curso
TEST FREE COURSE
curso
Introdução ao C++
curso
Noções Básicas de C#
projeto
Building a Classic Snake Game
curso
Fundamentos de C
projeto
Building a Classic Snake Game
curso
Fundamentos de C
curso
Introdução ao Python
curso
Introdução ao Python
curso
Introdução ao Python
curso
Introdução ao Python
curso
Introdução ao Python
curso
TEST FREE COURSE
curso
Introdução ao C++
curso
Noções Básicas de C#
projeto
Building a Classic Snake Game
curso
Fundamentos de C
projeto
Building a Classic Snake Game
curso
Fundamentos de C
Perguntas frequentes
Ainda tem dúvidas?
Escreva sua pergunta aqui