Nested while Loop
To summarize, the while
loop statement executes a code block repeatedly as long as a specific condition remains True
. We opt for a while
loop when the number of iterations isn't predetermined.
Now, let's delve into employing a while
loop within another while
loop.
Examine the code below:
How does the code work?
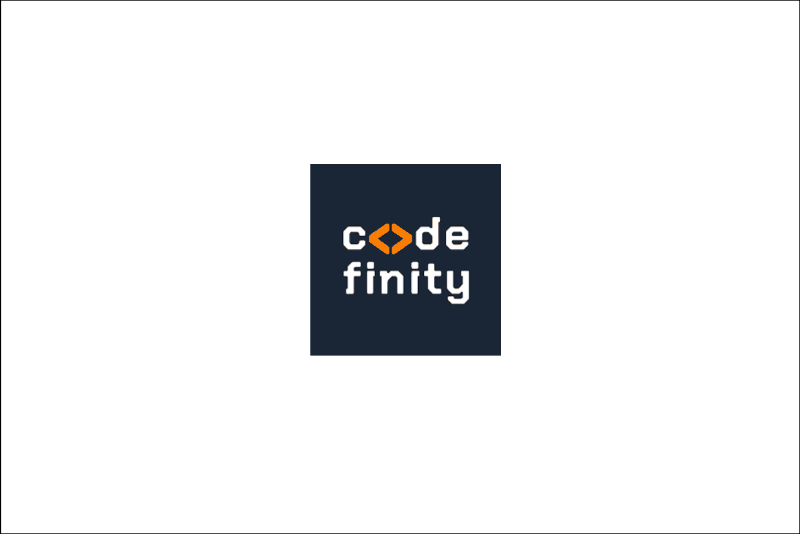
In the previous section, we worked with a matrix. We can achieve the same using a nested while
loop!
Examine the code below:
How does the code work?
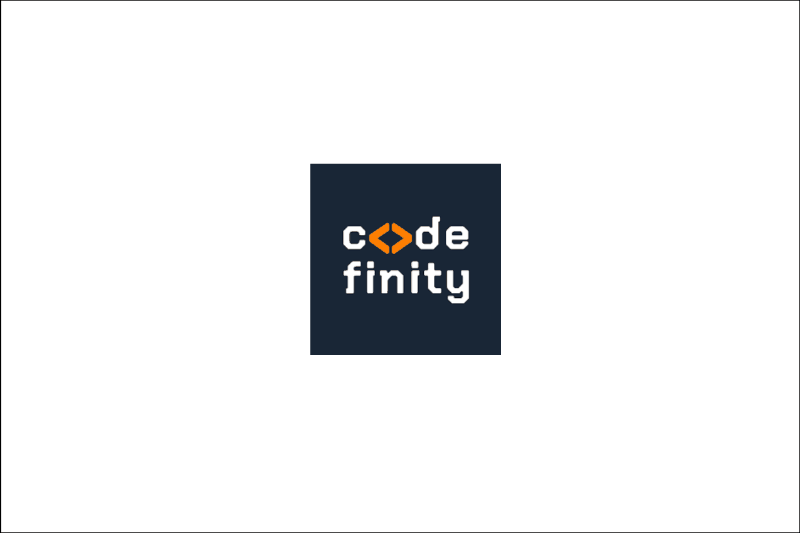
Task
Increment every element in the matrix by 1
.
- Configure the outer
while
loop to iterate through each row in the matrix. - Configure the inner
while
loop to iterate through each element in the row of the matrix. - Add
1
to every element. - Display each updated element.
Everything was clear?
Course Content
Python Loops Tutorial
Python Loops Tutorial
Nested while Loop
To summarize, the while
loop statement executes a code block repeatedly as long as a specific condition remains True
. We opt for a while
loop when the number of iterations isn't predetermined.
Now, let's delve into employing a while
loop within another while
loop.
Examine the code below:
How does the code work?
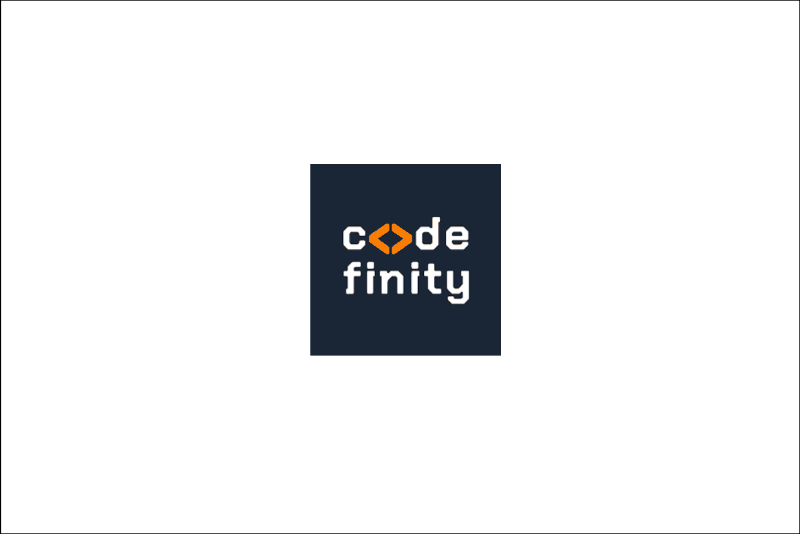
In the previous section, we worked with a matrix. We can achieve the same using a nested while
loop!
Examine the code below:
How does the code work?
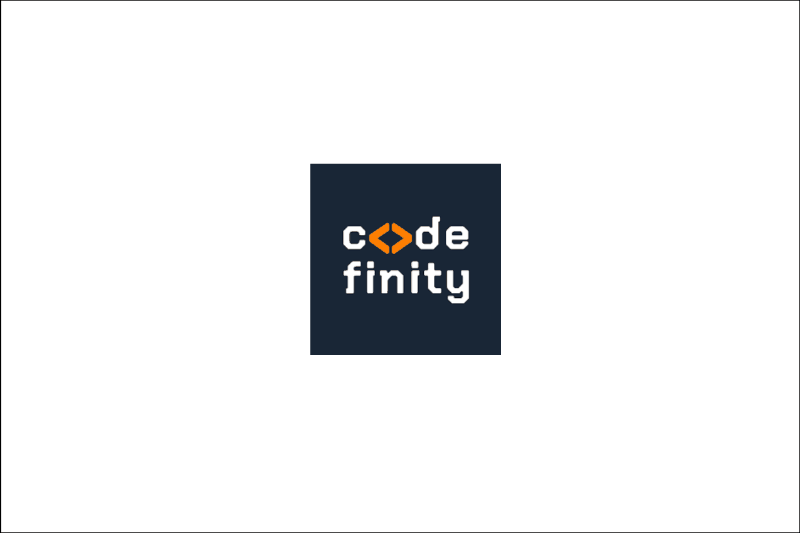
Task
Increment every element in the matrix by 1
.
- Configure the outer
while
loop to iterate through each row in the matrix. - Configure the inner
while
loop to iterate through each element in the row of the matrix. - Add
1
to every element. - Display each updated element.
Everything was clear?