Break/Continue in a Nested Loop
If the break
statement is employed within a nested loop, it will terminate the innermost loop.
Examine the code below:
How does the code work?
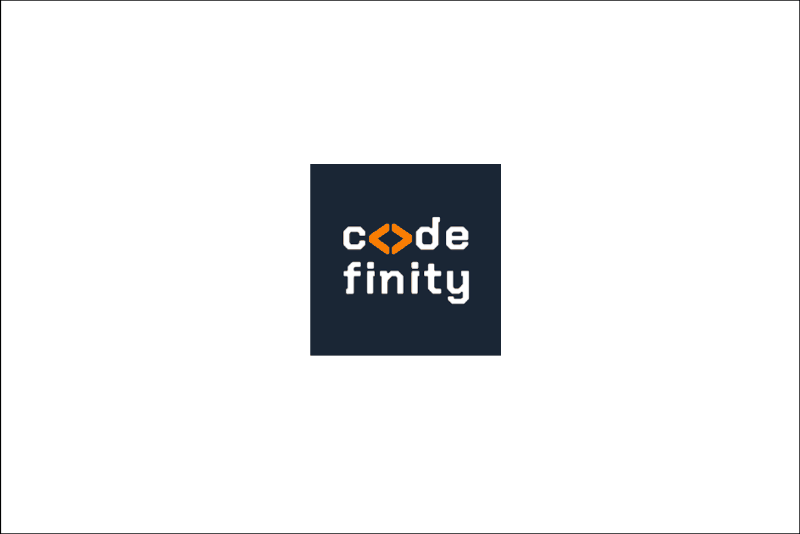
Please note that when the continue
statement is encountered inside the loop, it skips all the statements below it and immediately moves to the next iteration.
Examine the code below:
How does the code work?
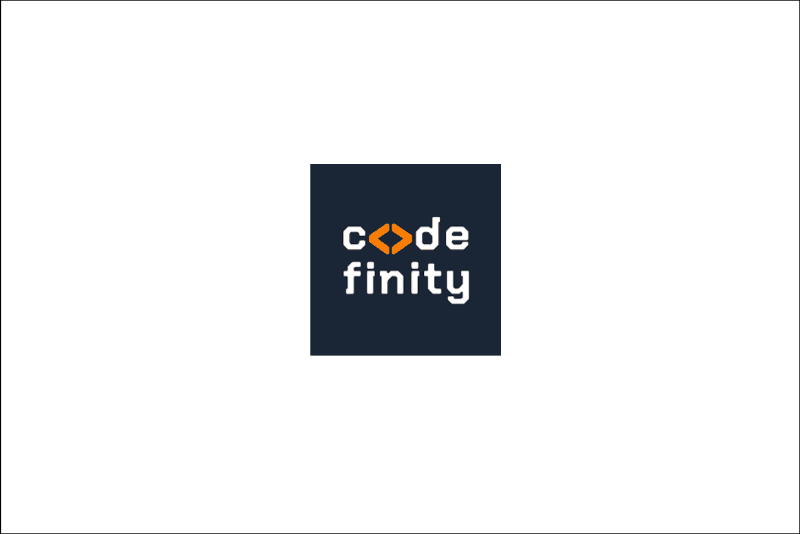
Task
You need to filter out symbols and numbers from the given list.
- Configure the outer
for
loop to iterate through the number of rows in the matrix. - Configure the inner
for
loop to iterate through the elements in each row of the matrix. - Implement the following conditions: If an element is
'#'
, thencontinue
the loop; else if an element is'!'
, thenbreak
out of the loop; if an element is neither'#'
nor'!'
, then print this element.
Everything was clear?
Course Content
Python Loops Tutorial
Python Loops Tutorial
Break/Continue in a Nested Loop
If the break
statement is employed within a nested loop, it will terminate the innermost loop.
Examine the code below:
How does the code work?
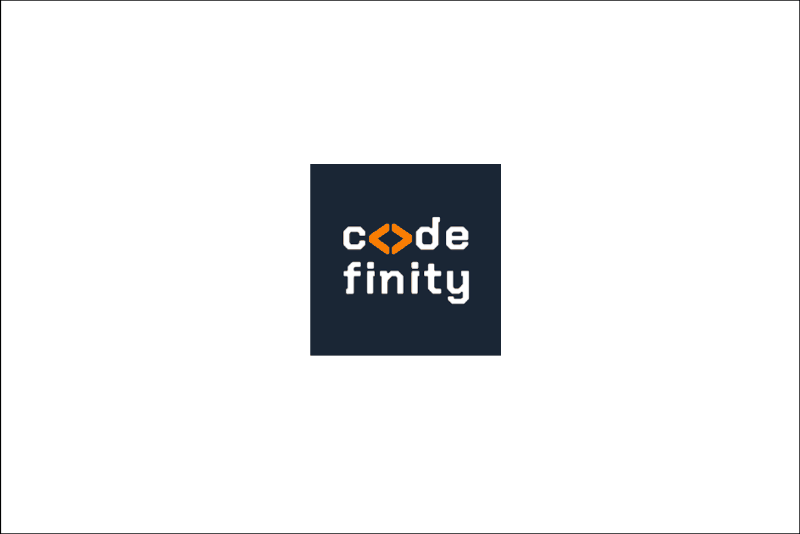
Please note that when the continue
statement is encountered inside the loop, it skips all the statements below it and immediately moves to the next iteration.
Examine the code below:
How does the code work?
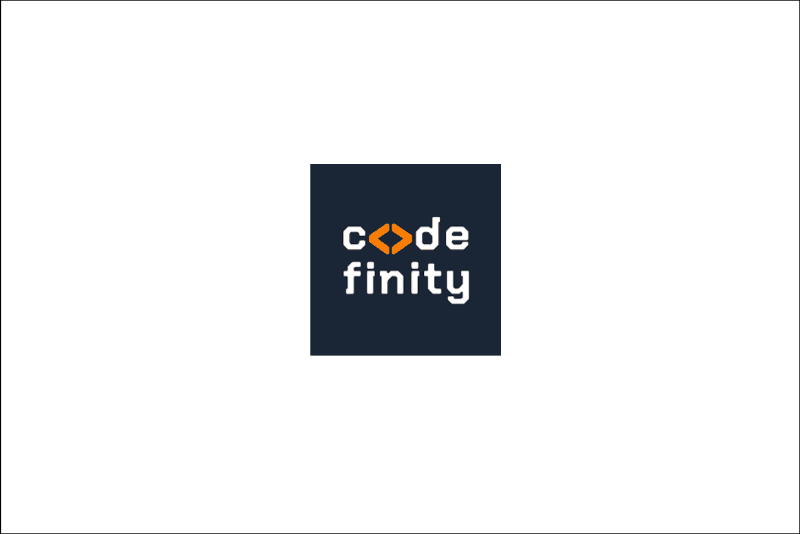
Task
You need to filter out symbols and numbers from the given list.
- Configure the outer
for
loop to iterate through the number of rows in the matrix. - Configure the inner
for
loop to iterate through the elements in each row of the matrix. - Implement the following conditions: If an element is
'#'
, thencontinue
the loop; else if an element is'!'
, thenbreak
out of the loop; if an element is neither'#'
nor'!'
, then print this element.
Everything was clear?