Contenido del Curso
C++ Loops
Introduction to Nested Loops
Nested loops, as the name suggests, are loops within loops. They allow you to create more complex and structured patterns of repetition. To understand this concept better, let's break it down:
- Outer loop: The outer loop is the main loop that controls the flow of your program. It's responsible for repeating the entire process multiple times;
- Inner loop(s): Inside the outer loop, you can have one or more inner loops. These inner loops have their own iteration control and can run multiple times before the outer loop progresses to the next iteration;
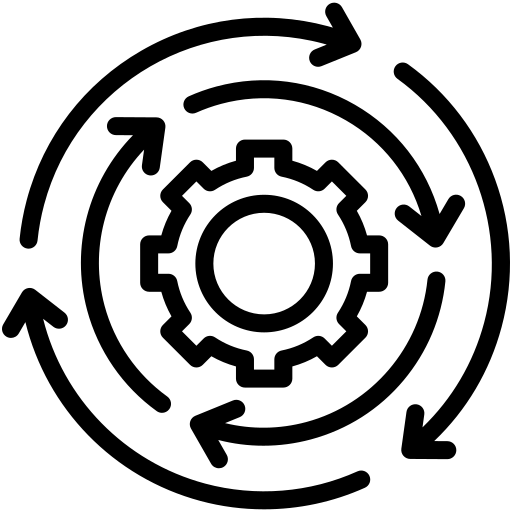
To illustrate nested loops, think about the process of marking all the apples inside multiple baskets.
- Outer Loop (Process of Taking a New Basket with Apples):
- Begin the process of taking a new basket;
- For each basket:
- Inner Loop (Process for Individual Apples in the Basket):
- Take an apple from the basket.
- Mark the apple;
- Put the marked apple back into the basket;
- Repeat these steps for every apple in the basket.
- Inner Loop (Process for Individual Apples in the Basket):
- End the process of taking a new basket.
¡Gracias por tus comentarios!