Course Content
Ultimate NumPy
3. Commonly used NumPy Functions
Ultimate NumPy
Sorting Arrays
Let’s start with perhaps the most common operation for arrays: sorting.
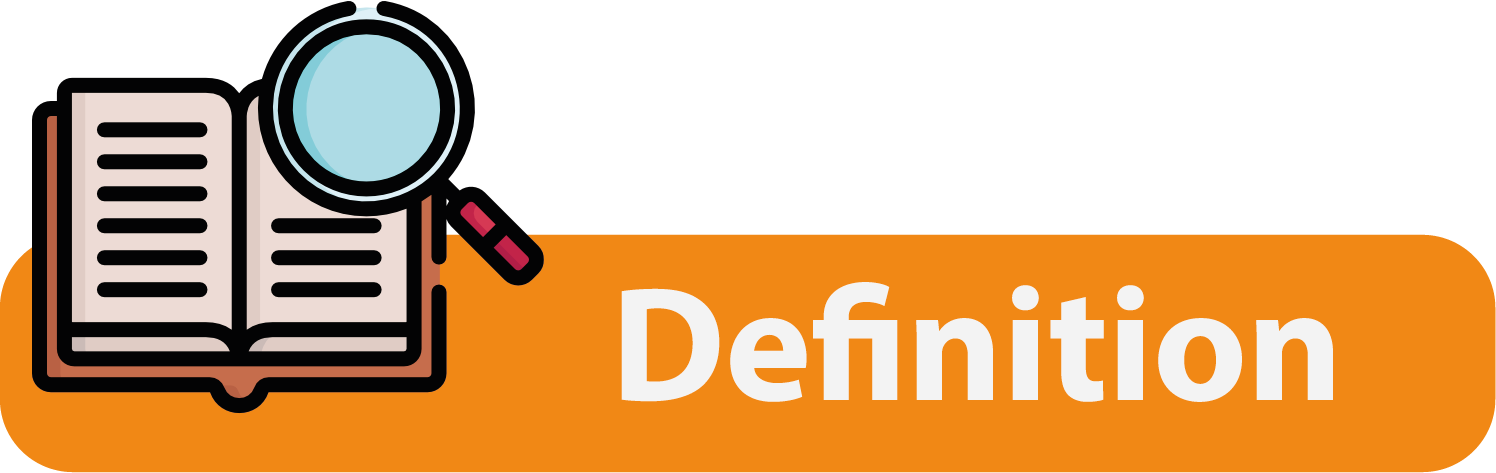
This is extremely useful for searching an element in an array since searching in a sorted array is much faster than in an unsorted one.
numpy.sort() function
NumPy has a built-in function sort()
for sorting elements by values in ascending order. The return value of this function is a sorted NumPy array. Here is its general syntax: numpy.sort(a, axis=-1, kind=None, order=None)
, where:
a
is our array;axis
is the axis along which to sort (last axis (-1
) by default);kind
is the sorting algorithm to use (quicksort
by default).
You will most likely rarely specify the kind
parameter and even less often the order
parameter, so we won’t discuss them here.
Let’s first have a look at an example with a 1D array:
Here is the visualization:
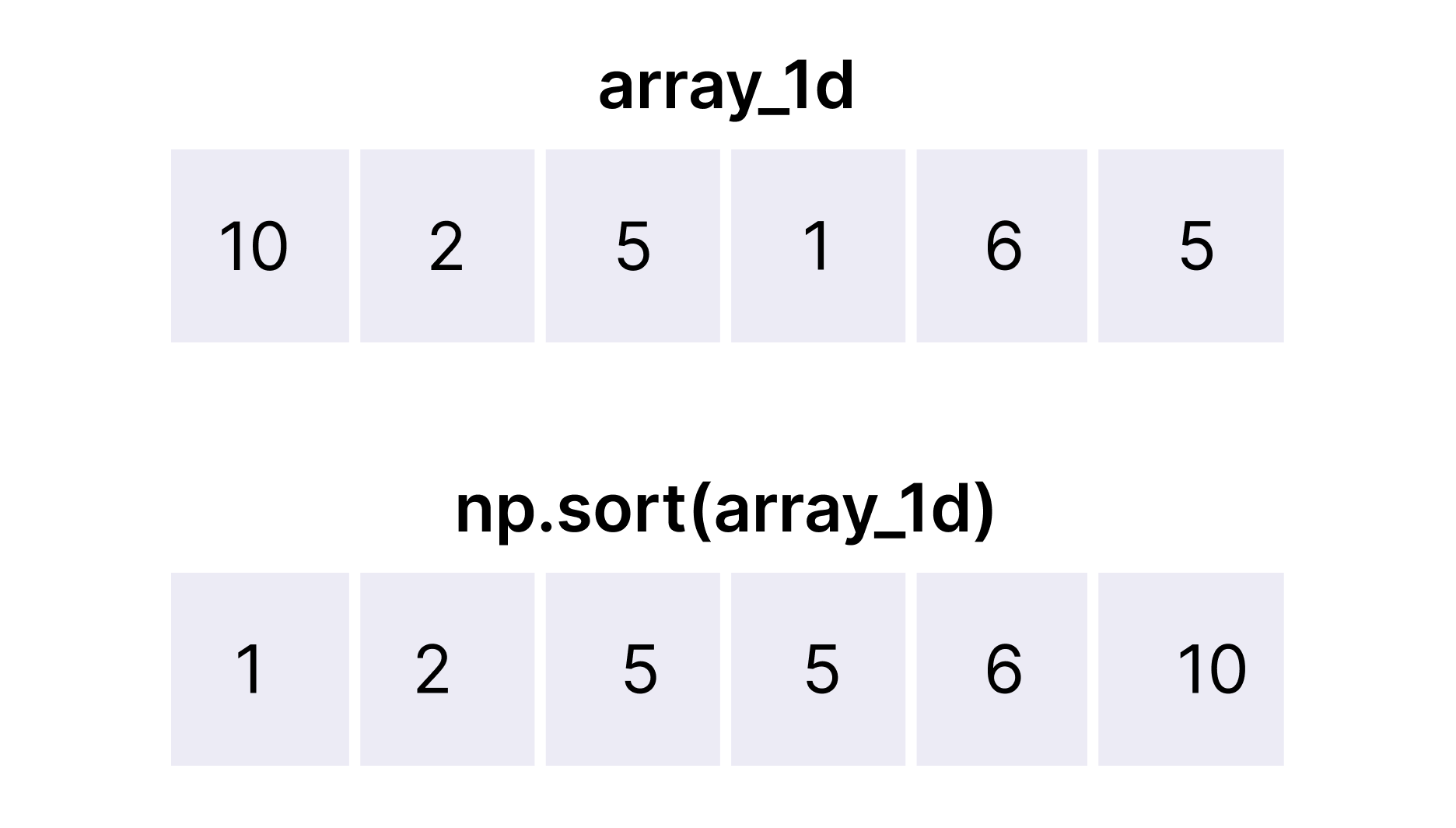
As you can see, with a 1D array, we only need to specify the a
parameter, and that’s enough.
ndarray.sort() method
As we already mentioned, the numpy.sort()
function returns a sorted array but does not change the original array. If we wanted to change the array, we would have to write array = np.sort(array)
.
However, NumPy has a .sort()
method as an alternative, which sorts the array in-place and returns None
. Its syntax is completely identical to the sort()
function.
Here is an example:
After calling the .sort()
method, array_1d
was sorted in place and now contains elements sorted in ascending order.
Sorting 1D Arrays in Descending Order
Sometimes we may want to sort an array in descending order. Neither the .sort()
method nor the sort()
function supports this functionality directly. However, we can simply use slicing with step
equal to -1
on a sorted array:
Let's take a look at the visualization:
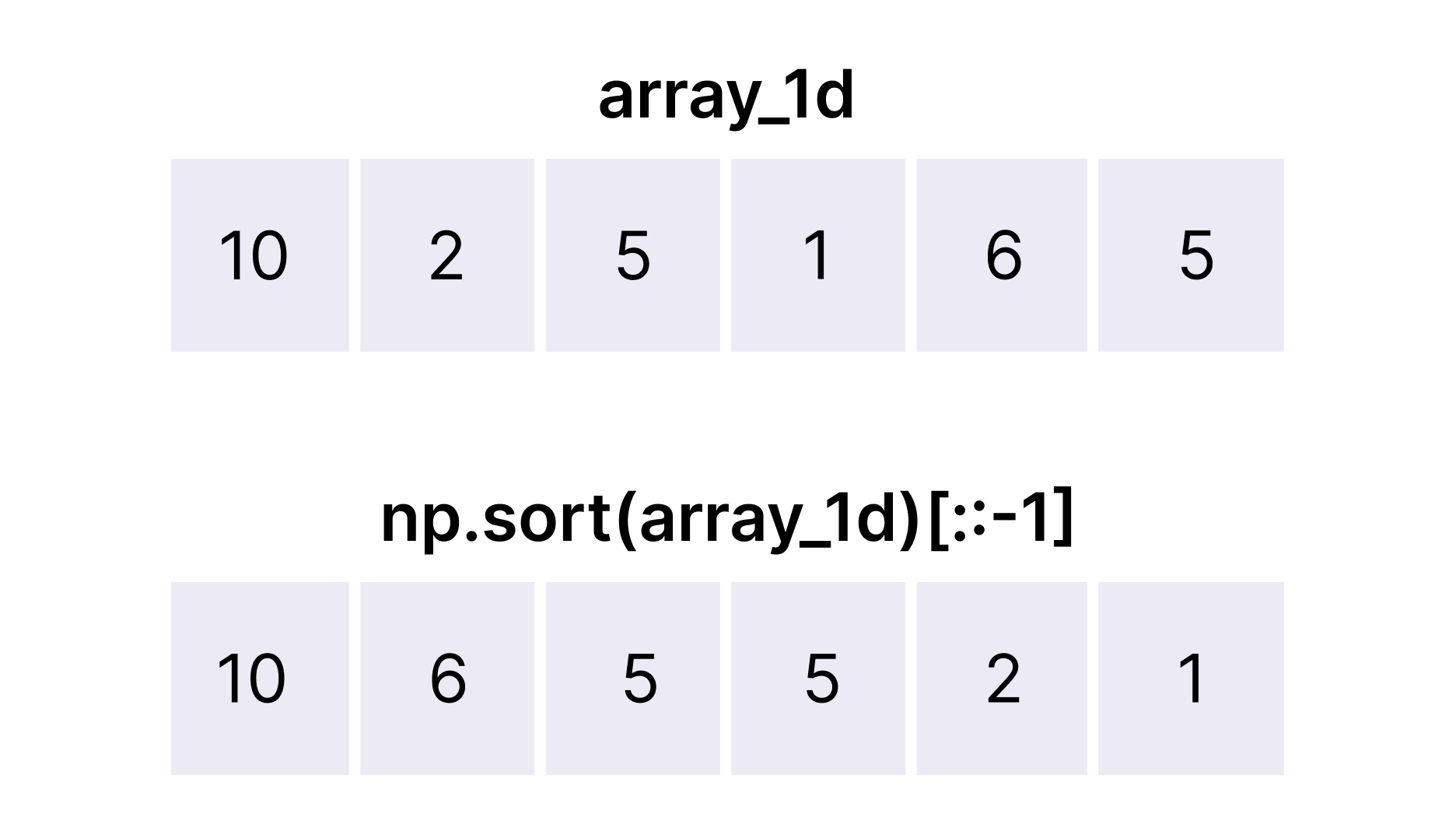
Task
You are managing a dataset of employee salaries stored in the salaries
array. Your task is the following:
- Sort the salaries in descending order using the appropriate function.
- Print the top 3 salaries using a slice and specifying only a positive
end
.
Everything was clear?
Course Content
Ultimate NumPy
3. Commonly used NumPy Functions
Ultimate NumPy
Sorting Arrays
Let’s start with perhaps the most common operation for arrays: sorting.
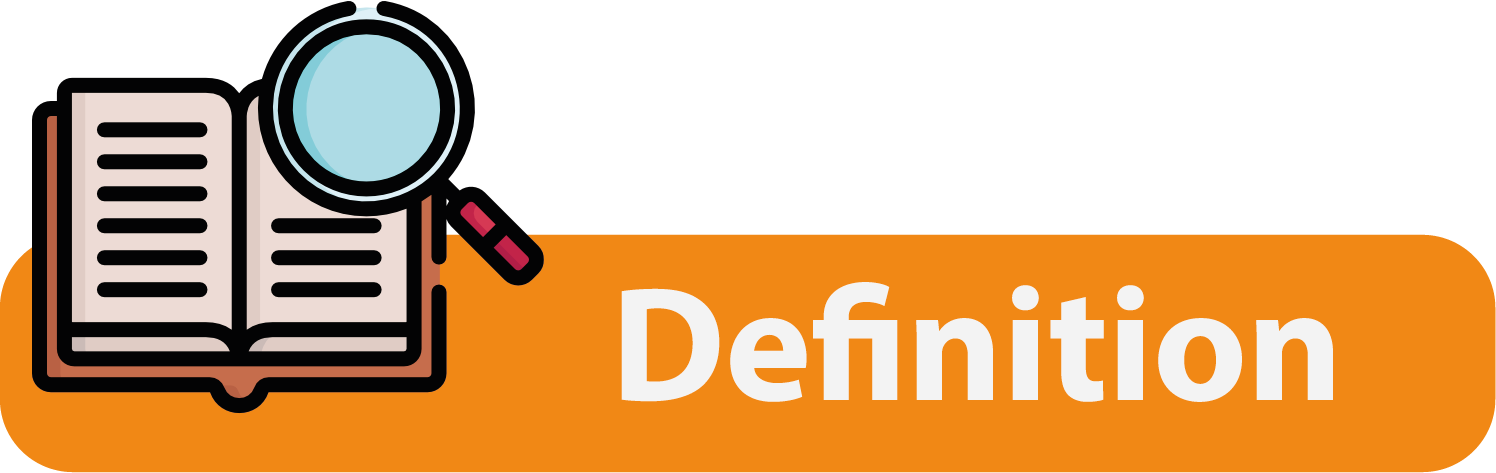
This is extremely useful for searching an element in an array since searching in a sorted array is much faster than in an unsorted one.
numpy.sort() function
NumPy has a built-in function sort()
for sorting elements by values in ascending order. The return value of this function is a sorted NumPy array. Here is its general syntax: numpy.sort(a, axis=-1, kind=None, order=None)
, where:
a
is our array;axis
is the axis along which to sort (last axis (-1
) by default);kind
is the sorting algorithm to use (quicksort
by default).
You will most likely rarely specify the kind
parameter and even less often the order
parameter, so we won’t discuss them here.
Let’s first have a look at an example with a 1D array:
Here is the visualization:
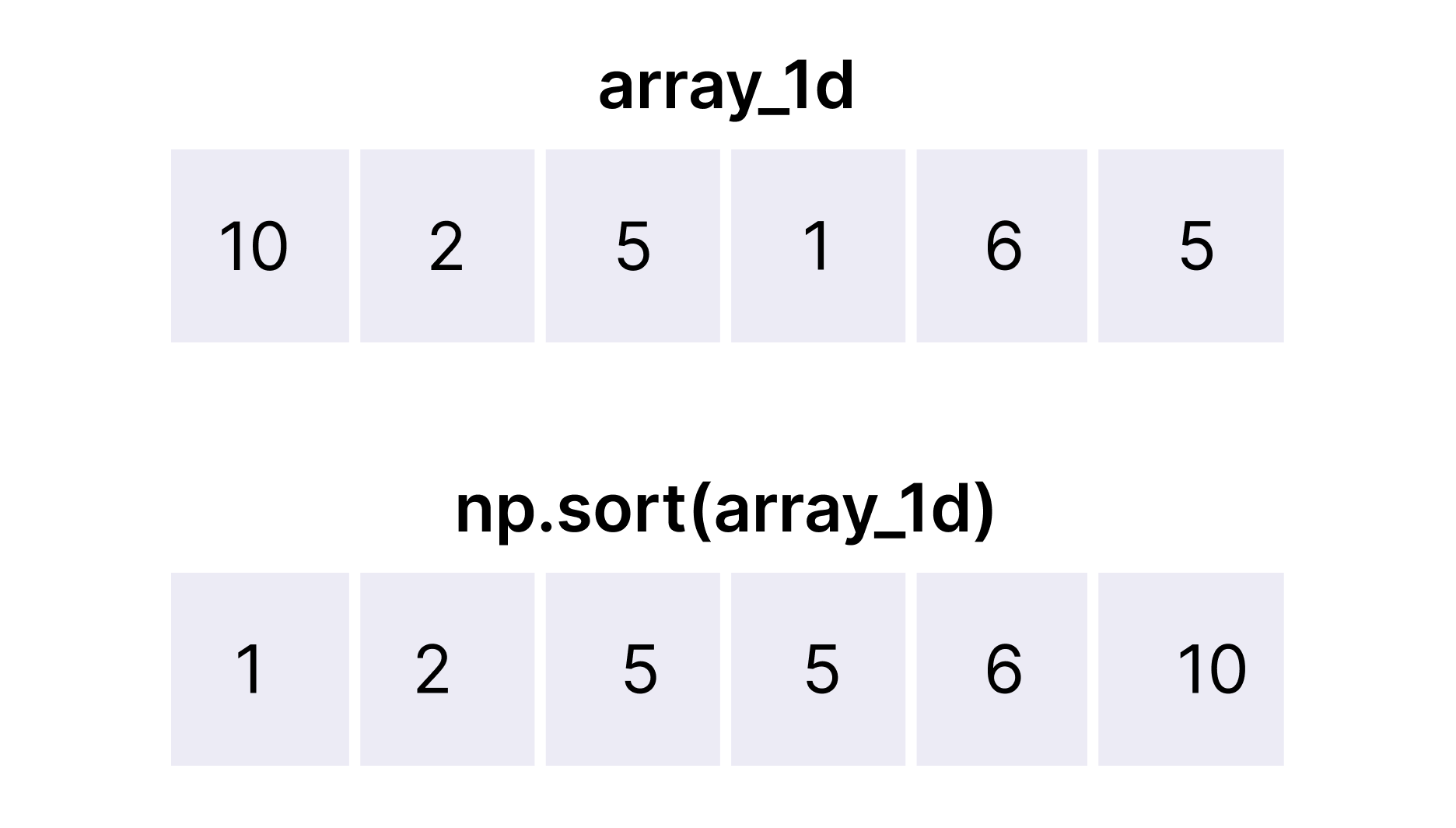
As you can see, with a 1D array, we only need to specify the a
parameter, and that’s enough.
ndarray.sort() method
As we already mentioned, the numpy.sort()
function returns a sorted array but does not change the original array. If we wanted to change the array, we would have to write array = np.sort(array)
.
However, NumPy has a .sort()
method as an alternative, which sorts the array in-place and returns None
. Its syntax is completely identical to the sort()
function.
Here is an example:
After calling the .sort()
method, array_1d
was sorted in place and now contains elements sorted in ascending order.
Sorting 1D Arrays in Descending Order
Sometimes we may want to sort an array in descending order. Neither the .sort()
method nor the sort()
function supports this functionality directly. However, we can simply use slicing with step
equal to -1
on a sorted array:
Let's take a look at the visualization:
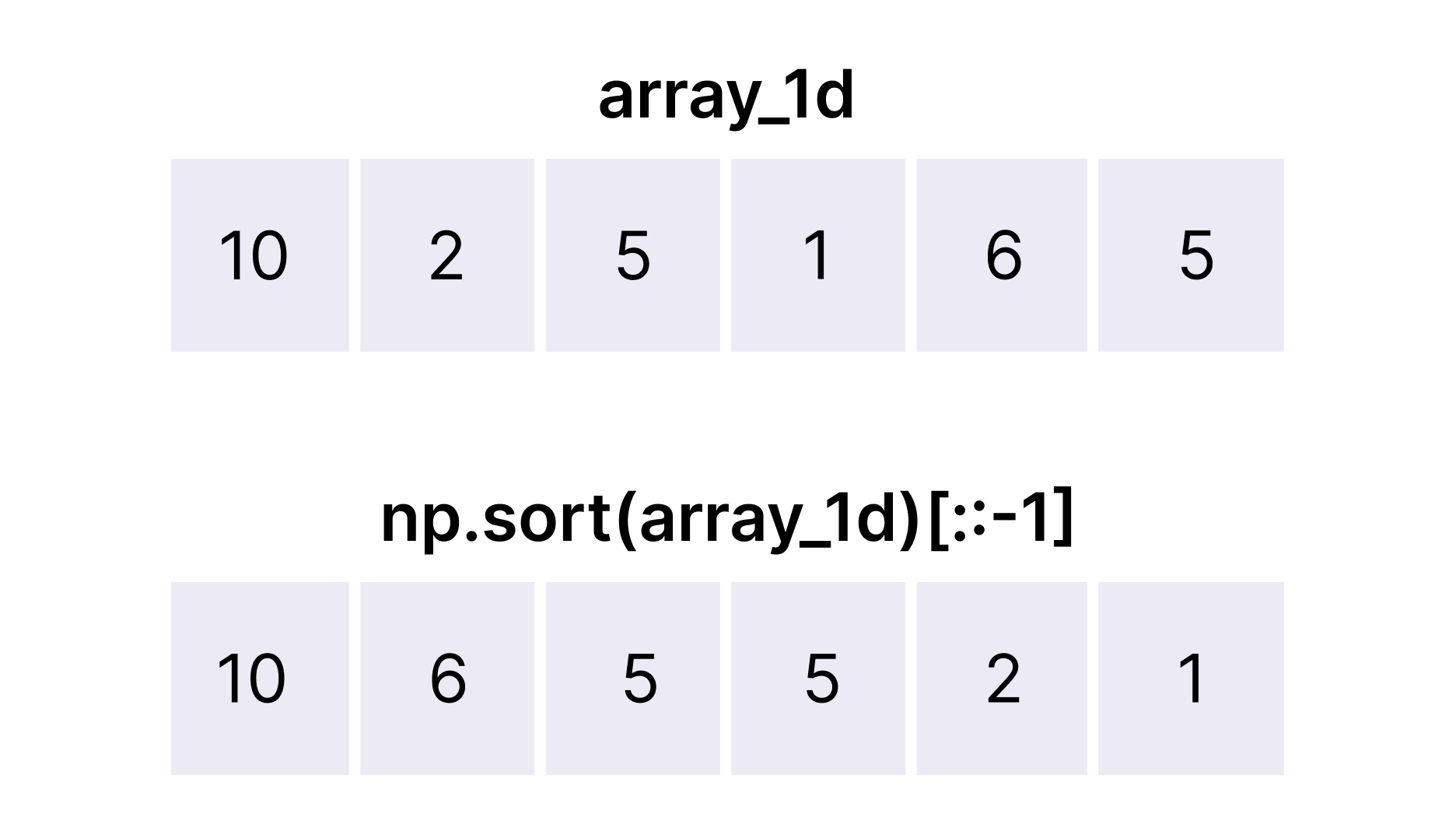
Task
You are managing a dataset of employee salaries stored in the salaries
array. Your task is the following:
- Sort the salaries in descending order using the appropriate function.
- Print the top 3 salaries using a slice and specifying only a positive
end
.
Everything was clear?