Desafio: Usando iloc
O DataFrame com o qual estamos trabalhando:
Você também pode usar indexação negativa para acessar linhas no DataFrame. A indexação negativa começa do final do DataFrame: o índice -1
aponta para a última linha, -2
para a penúltima, e assim por diante.
Para acessar a sétima linha (que se refere à Letônia), você pode usar o índice 6 ou -1.
import pandas countries_data = {'country' : ['Thailand', 'Philippines', 'Monaco', 'Malta', 'Sweden', 'Paraguay', 'Latvia'], 'continent' : ['Asia', 'Asia', 'Europe', 'Europe', 'Europe', 'South America', 'Europe'], 'capital':['Bangkok', 'Manila', 'Monaco', 'Valletta', 'Stockholm', 'Asuncion', 'Riga']} countries = pandas.DataFrame(countries_data) # Accessing to the seventh row using negative indexing print(countries.iloc[-1])
Executar o código acima retornará a linha destacada na imagem abaixo:
Tarefa
Swipe to start coding
- Exiba todos os detalhes do DataFrame para o modelo
Audi A1
do ano de 2017. Para fazer isso, você precisará usar indexação positiva. - Exiba todos os detalhes do DataFrame para o modelo
Audi A1
do ano de 2016 usando indexação negativa. - Exiba todos os detalhes do DataFrame para o modelo
Audi A3
usando indexação positiva.
Certifique-se de usar o atributo iloc
.
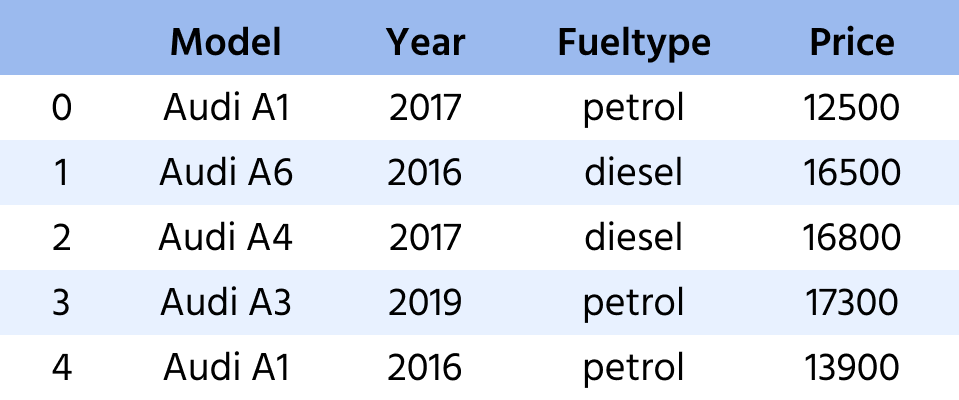
Solução
Tudo estava claro?
Obrigado pelo seu feedback!
Seção 1. Capítulo 14