Contenido del Curso
Introduction to TensorFlow
Introduction to TensorFlow
Basic Operations: Linear Algebra
Linear Algebra Operations
TensorFlow offers a suite of functions dedicated to linear algebra operations, making matrix operations straightforward.
Matrix Multiplication
Here's a quick reminder of how matrix multiplication works.
There are two equivalent approaches for matrix multiplication:
- The
tf.matmul()
function; - Using the
@
operator.
import tensorflow as tf # Create two matrices matrix1 = tf.constant([[1, 2], [3, 4], [2, 1]]) matrix2 = tf.constant([[2, 0, 2, 5], [2, 2, 1, 3]]) # Multiply the matrices product1 = tf.matmul(matrix1, matrix2) product2 = matrix1 @ matrix2 # Display tensors print(product1) print('-' * 50) print(product2)
Note
Multiplying matrices of size 3x2 and 2x4 will give a matrix of 3x4.
Matrix Inversion
You can obtain the inverse of a matrix using the tf.linalg.inv()
function. Additionally, let's verify a fundamental property of the inverse matrix.
import tensorflow as tf # Create 2x2 matrix matrix = tf.constant([[1., 2.], [3., 4.]]) # Compute the inverse of a matrix inverse_mat = tf.linalg.inv(matrix) # Check the result identity = matrix @ inverse_mat # Display tensors print(inverse_mat) print('-' * 50) print(identity)
Note
Multiplying a matrix with its inverse should yield an identity matrix, which has ones on its main diagonal and zeros everywhere else. Additionally, the
tf.linalg
module offers a wide range of linear algebra functions. For further details or more advanced operations, you might want to refer to its official documentation.
Transpose
You can obtain a transposed matrix using the tf.transpose()
function.
import tensorflow as tf # Create a matrix 3x2 matrix = tf.constant([[1, 2], [3, 4], [2, 1]]) # Get the transpose of a matrix transposed = tf.transpose(matrix) # Display tensors print(matrix) print('-' * 40) print(transposed)
Dot Product
You can obtain a dot product using the tf.tensordot()
function. By setting up an axes argument you can choose along which axes to calculate a dot product. E.g. for two vectors by setting up axes=1
you will get the classic dot product between vectors. But when setting axes=0
you will get broadcasted matrix along 0 axes:
import tensorflow as tf # Create two vectors matrix1 = tf.constant([1, 2, 3, 4]) matrix2 = tf.constant([2, 0, 2, 5]) # Compute the dot product of two tensors dot_product_axes1 = tf.tensordot(matrix1, matrix2, axes=1) dot_product_axes0 = tf.tensordot(matrix1, matrix2, axes=0) # Display tensors print(dot_product_axes1) print('-' * 40) print(dot_product_axes0)
Note
If you take two matrices with appropriate dimensions (
NxM @ MxK
, whereNxM
represents the dimensions of the first matrix andMxK
the second), and compute the dot product alongaxes=1
, it essentially performs matrix multiplication.
Swipe to start coding
Background
A system of linear equations can be represented in matrix form using the equation:
AX = B
Where:
A
is a matrix of coefficients.X
is a column matrix of variables.B
is a column matrix representing the values on the right side of the equations.
The solution to this system can be found using the formula:
X = A^-1 B
Where A^-1
is the inverse of matrix A
.
Objective
Given a system of linear equations, use TensorFlow to solve it. You are given the following system of linear equations:
2x + 3y - z = 1
.4x + y + 2z = 2
.-x + 2y + 3z = 3
.
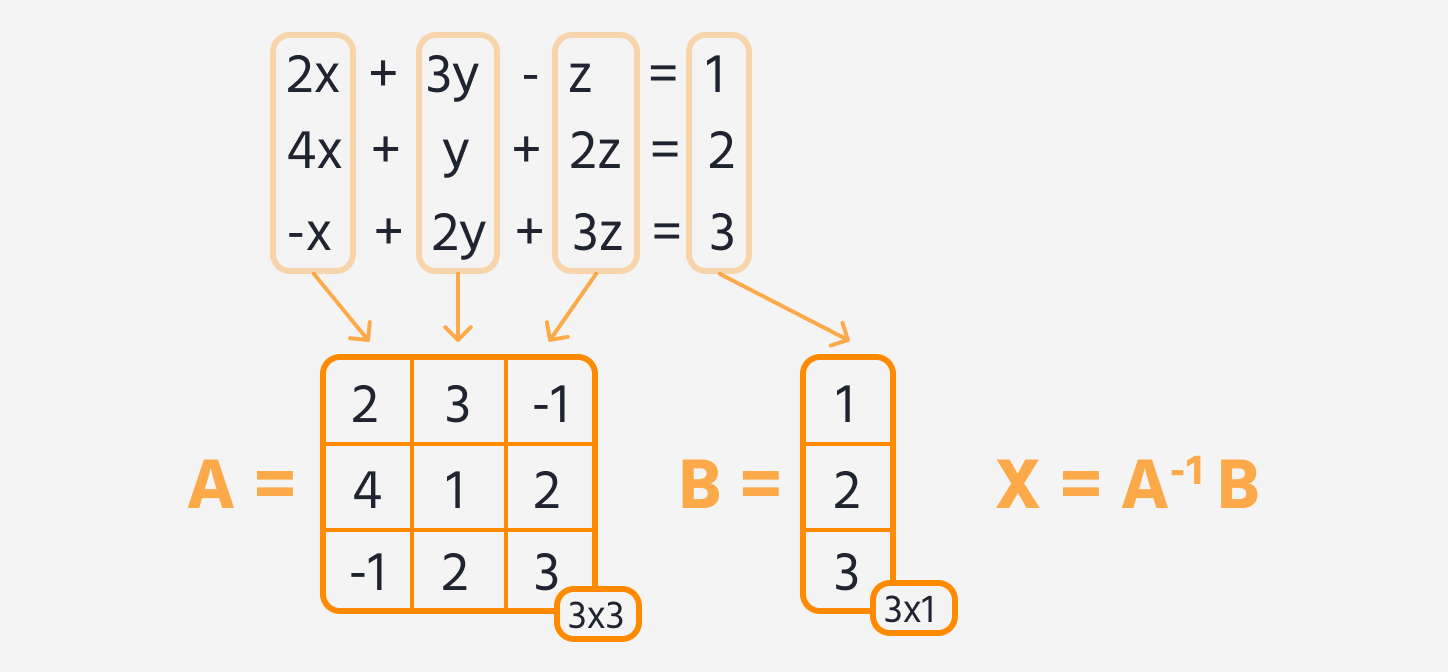
- Represent the system of equations in matrix form (separate it into matrices
A
andB
). - Using TensorFlow, find the inverse of matrix
A
. - Multiply the inverse of matrix
A
by matrixB
to find the solution matrixX
, which contains the values ofx
,y
, andz
.
Note
Slicing in TensorFlow operates similarly to NumPy. Therefore,
X[:, 0]
will retrieve all elements from the column at index0
. We will get to the slicing later in the course.
Solución
¡Gracias por tus comentarios!
Basic Operations: Linear Algebra
Linear Algebra Operations
TensorFlow offers a suite of functions dedicated to linear algebra operations, making matrix operations straightforward.
Matrix Multiplication
Here's a quick reminder of how matrix multiplication works.
There are two equivalent approaches for matrix multiplication:
- The
tf.matmul()
function; - Using the
@
operator.
import tensorflow as tf # Create two matrices matrix1 = tf.constant([[1, 2], [3, 4], [2, 1]]) matrix2 = tf.constant([[2, 0, 2, 5], [2, 2, 1, 3]]) # Multiply the matrices product1 = tf.matmul(matrix1, matrix2) product2 = matrix1 @ matrix2 # Display tensors print(product1) print('-' * 50) print(product2)
Note
Multiplying matrices of size 3x2 and 2x4 will give a matrix of 3x4.
Matrix Inversion
You can obtain the inverse of a matrix using the tf.linalg.inv()
function. Additionally, let's verify a fundamental property of the inverse matrix.
import tensorflow as tf # Create 2x2 matrix matrix = tf.constant([[1., 2.], [3., 4.]]) # Compute the inverse of a matrix inverse_mat = tf.linalg.inv(matrix) # Check the result identity = matrix @ inverse_mat # Display tensors print(inverse_mat) print('-' * 50) print(identity)
Note
Multiplying a matrix with its inverse should yield an identity matrix, which has ones on its main diagonal and zeros everywhere else. Additionally, the
tf.linalg
module offers a wide range of linear algebra functions. For further details or more advanced operations, you might want to refer to its official documentation.
Transpose
You can obtain a transposed matrix using the tf.transpose()
function.
import tensorflow as tf # Create a matrix 3x2 matrix = tf.constant([[1, 2], [3, 4], [2, 1]]) # Get the transpose of a matrix transposed = tf.transpose(matrix) # Display tensors print(matrix) print('-' * 40) print(transposed)
Dot Product
You can obtain a dot product using the tf.tensordot()
function. By setting up an axes argument you can choose along which axes to calculate a dot product. E.g. for two vectors by setting up axes=1
you will get the classic dot product between vectors. But when setting axes=0
you will get broadcasted matrix along 0 axes:
import tensorflow as tf # Create two vectors matrix1 = tf.constant([1, 2, 3, 4]) matrix2 = tf.constant([2, 0, 2, 5]) # Compute the dot product of two tensors dot_product_axes1 = tf.tensordot(matrix1, matrix2, axes=1) dot_product_axes0 = tf.tensordot(matrix1, matrix2, axes=0) # Display tensors print(dot_product_axes1) print('-' * 40) print(dot_product_axes0)
Note
If you take two matrices with appropriate dimensions (
NxM @ MxK
, whereNxM
represents the dimensions of the first matrix andMxK
the second), and compute the dot product alongaxes=1
, it essentially performs matrix multiplication.
Swipe to start coding
Background
A system of linear equations can be represented in matrix form using the equation:
AX = B
Where:
A
is a matrix of coefficients.X
is a column matrix of variables.B
is a column matrix representing the values on the right side of the equations.
The solution to this system can be found using the formula:
X = A^-1 B
Where A^-1
is the inverse of matrix A
.
Objective
Given a system of linear equations, use TensorFlow to solve it. You are given the following system of linear equations:
2x + 3y - z = 1
.4x + y + 2z = 2
.-x + 2y + 3z = 3
.
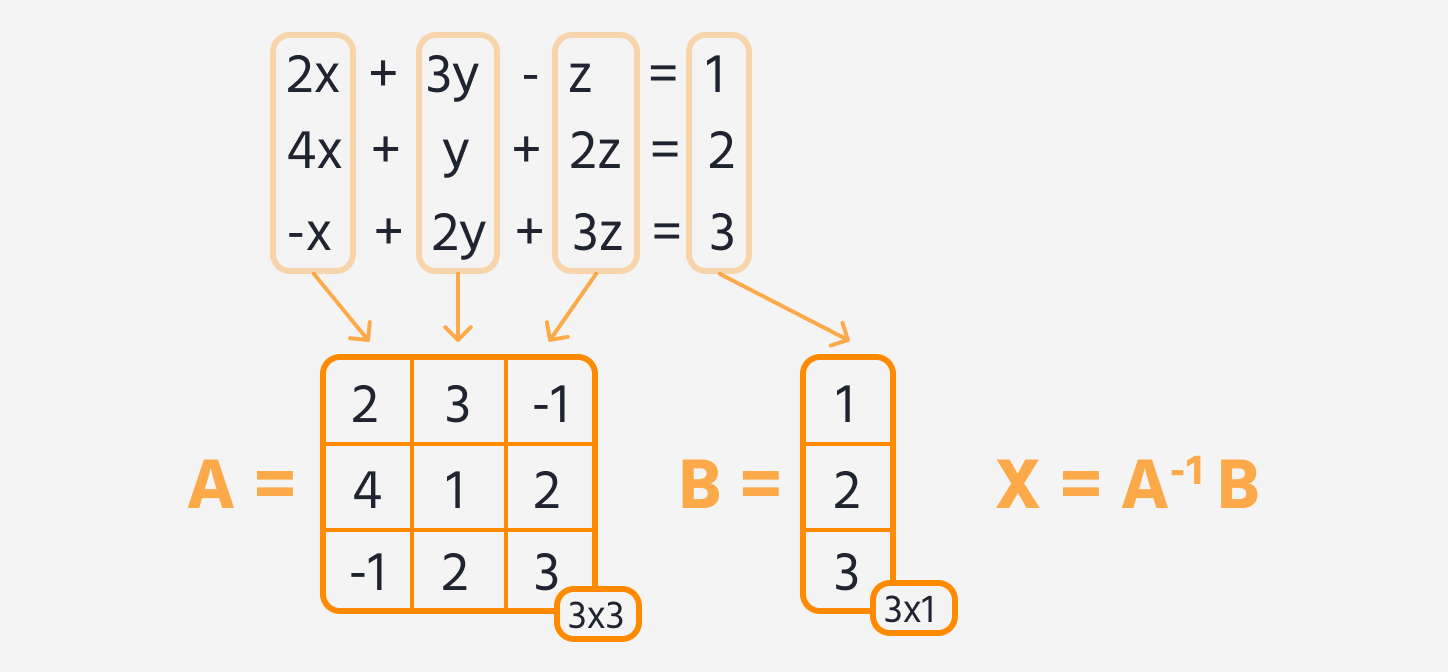
- Represent the system of equations in matrix form (separate it into matrices
A
andB
). - Using TensorFlow, find the inverse of matrix
A
. - Multiply the inverse of matrix
A
by matrixB
to find the solution matrixX
, which contains the values ofx
,y
, andz
.
Note
Slicing in TensorFlow operates similarly to NumPy. Therefore,
X[:, 0]
will retrieve all elements from the column at index0
. We will get to the slicing later in the course.
Solución
¡Gracias por tus comentarios!